PHP templating walkthrough: Part 2
Introduction
The whole point of PHP is to get the computer to do the busywork. It’s hard to see the purpose with just some simple little Madlib or exercises with very little data.
To see how useful it is, pretend you have a huge dataset. In this case, we’ll use guitars. We’ll just show 3, but pretend there are 3000 guitars.
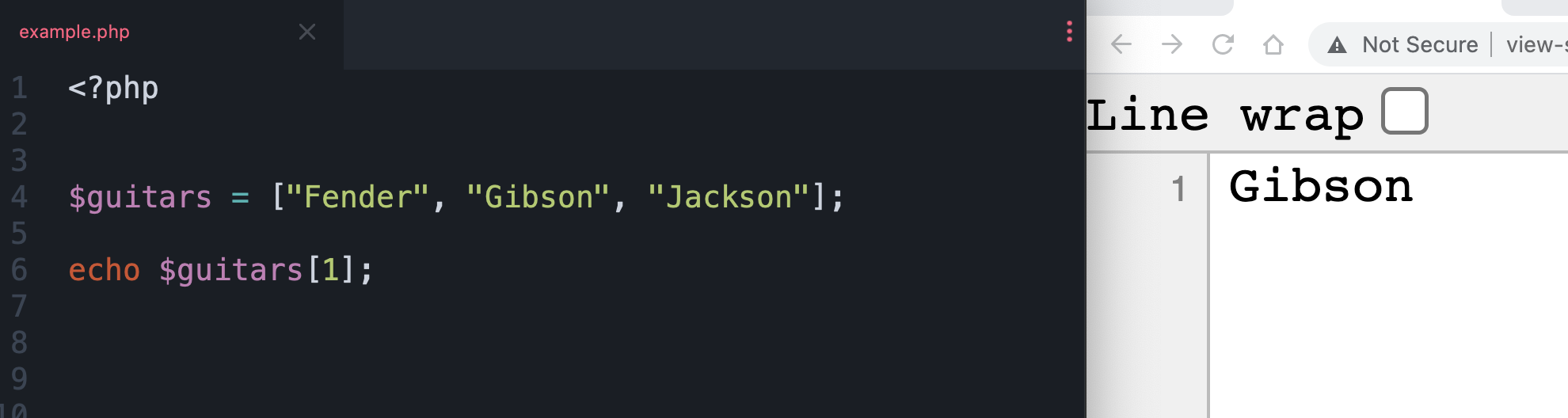
Here is an Array of Strings. “Strings” of characters.
PHP doesn’t know what they are. They could be anything. It doesn’t matter. What matters, is that strings are strings and variables are variables. Also, there’s this concept of the array – and that’s denoted with these square brackets. The items in the array are separated by commas.
To access an item in an array, you can dig a level deeper into the reference (variable) with what is called “Bracket notation.” Behind the scenes, each item has an “index” number to keep their order. You can use this number to pluck out the item of your choosing. But as you know, the numbers start at 0.
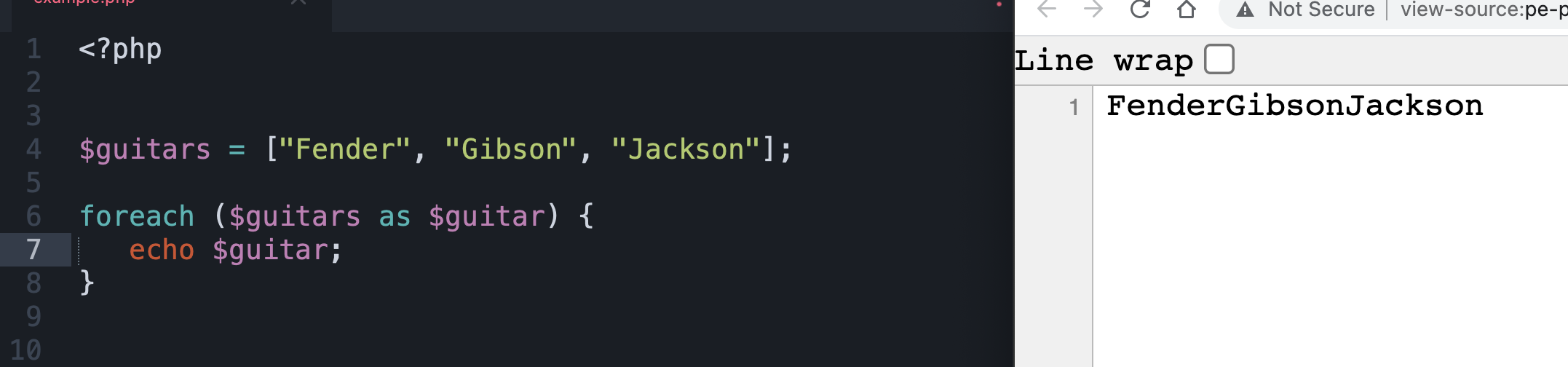
To iterate (loop) over a list (array) of items, you can use the foreach
construct. It will run a specified block of code for every item that exists in the array. This array has 3 items, so it will run the code 3 times.
The foreach takes some iterable (loopable/list/object) and then on each iteration, assigns the value of that item to a variable of your choosing. It could have been anything, but we chose $guitar
because that’s easy to understand. Plural for the list and singular for the item seems to be the convention.
https://www.php.net/manual/en/control-structures.foreach.php
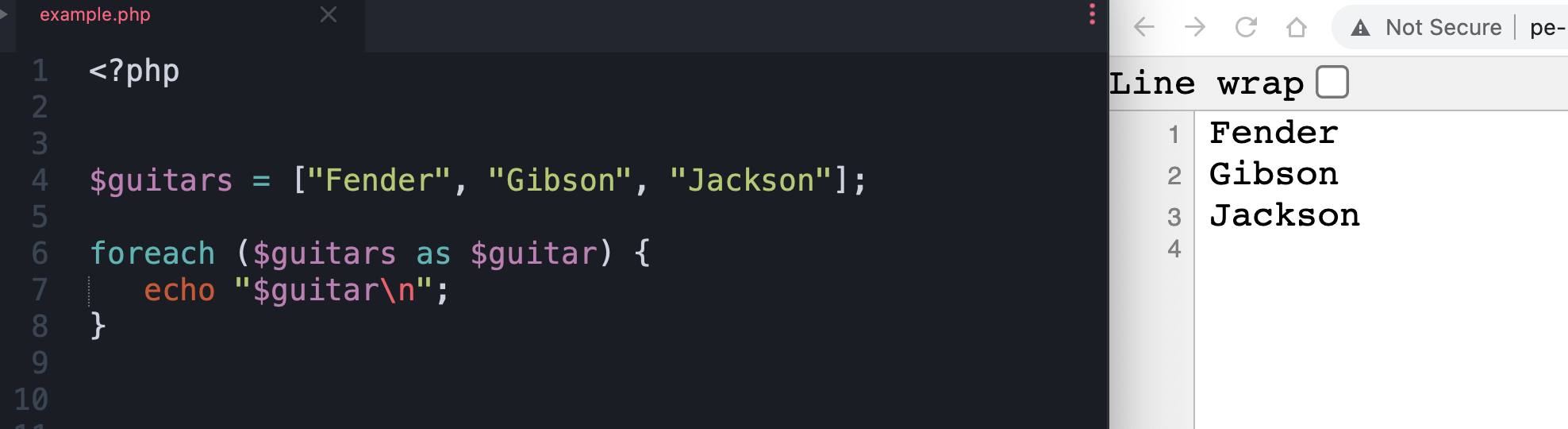
You can create a line break with the mysterious “Backslash.”
However, we find very few reasons to do so. It’s good to know how though!
Think “weird slash + n
for new line.”
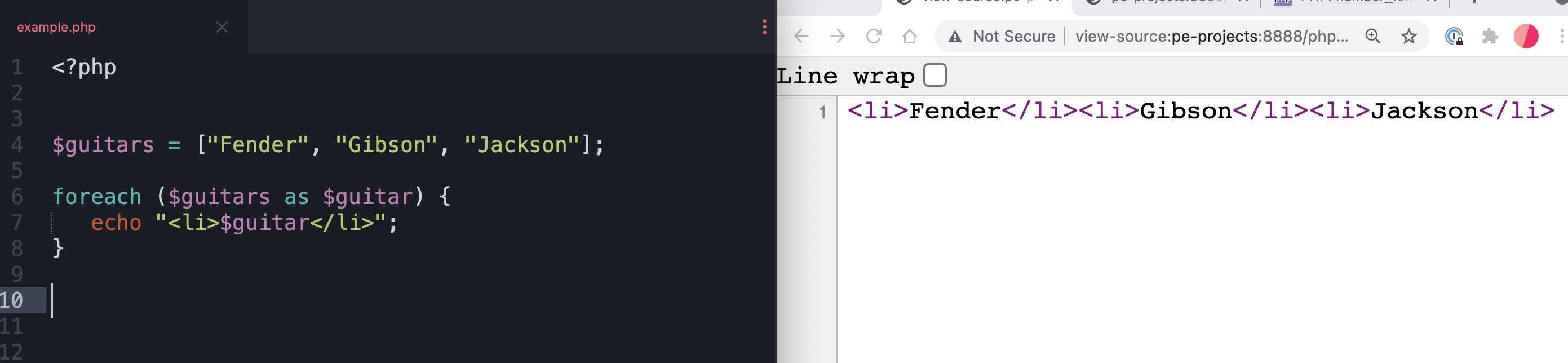
Right?
If you’re just working out ideas, it’s ok to use the li
and skip the ul
or ol
. The browser will just deal with it.
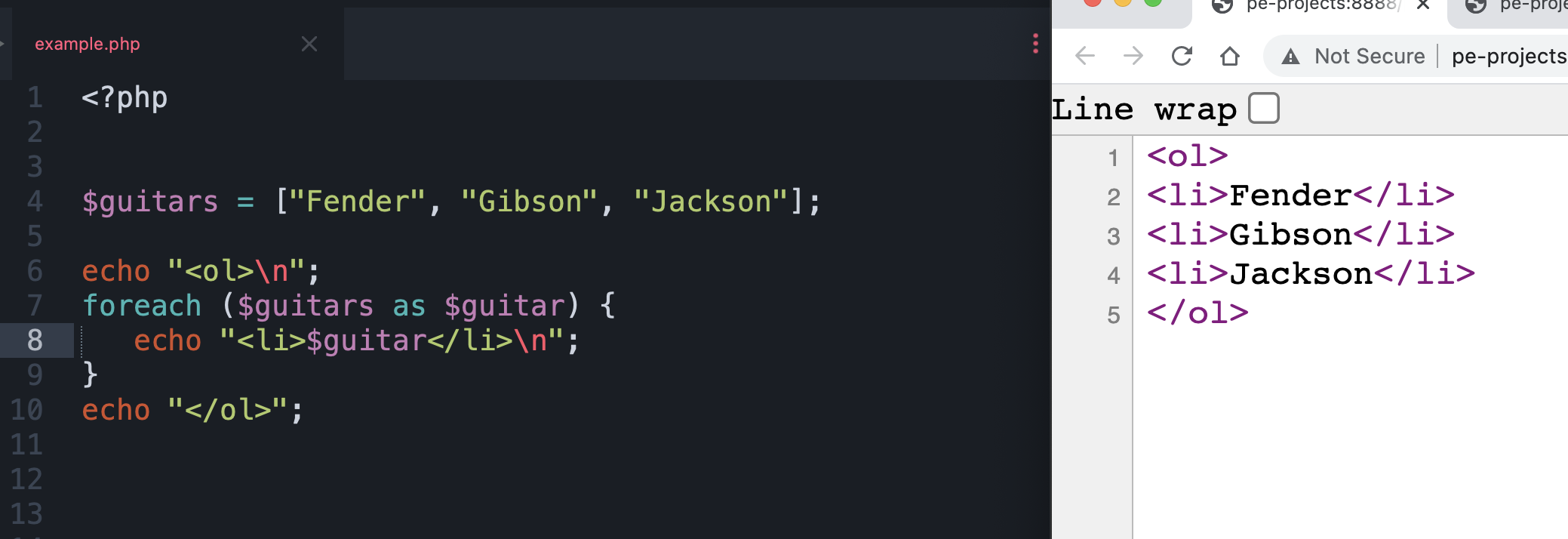
You can try and keep it looking nice, but eventually – you’ll have to let go of that idea. Please don’t obsess about how the final HTML looks. Unless you can figure out how to make it always look perfect: then – please tell us how.
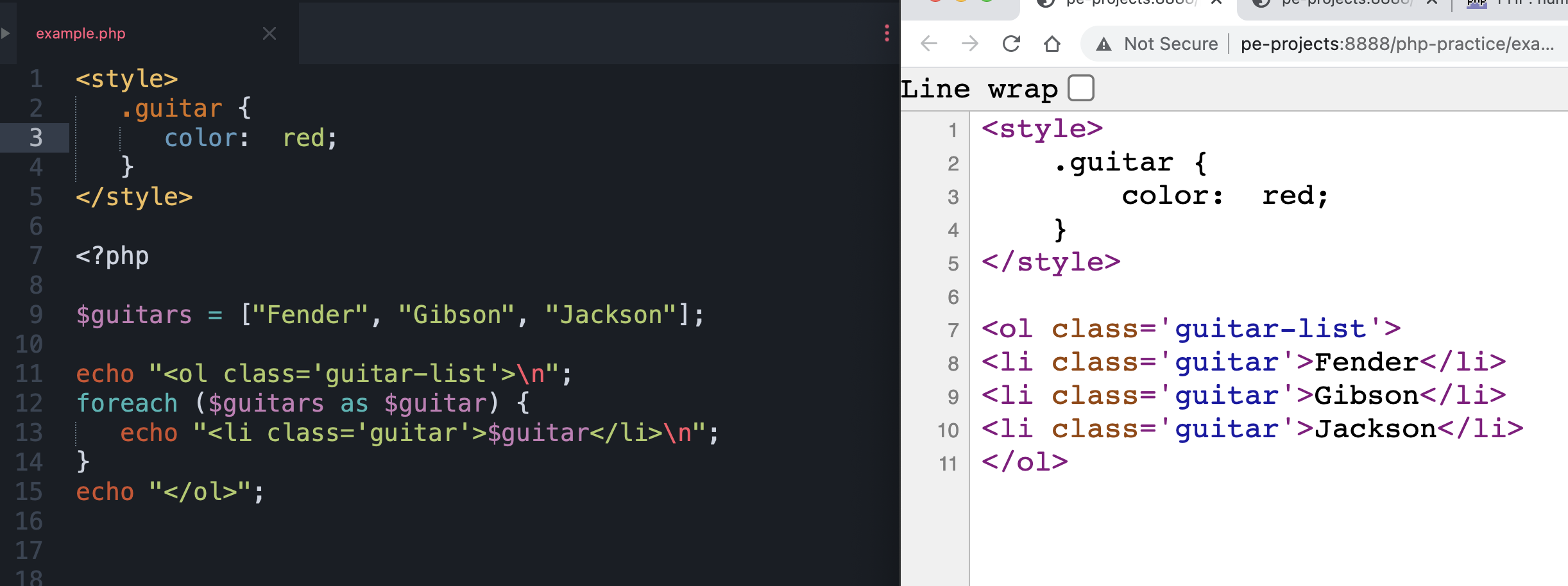
If you want to toss in some quick styles, you can use the style information element. Remember that one? Normally it belongs in the head
but you can use it here to mock out ideas.
You can just trust us that the text will turn red. Too many screenshots! We can’t put all of them here 😉
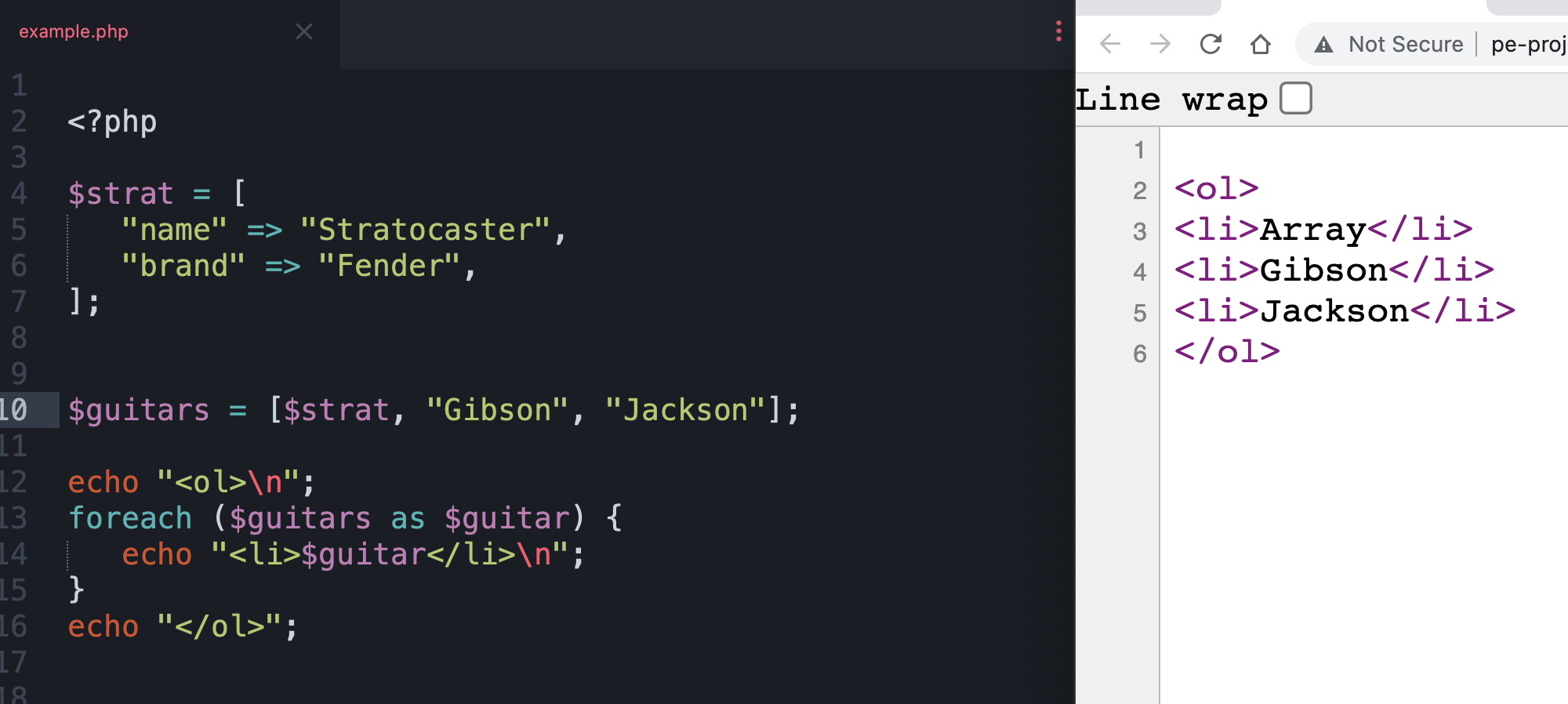
Most data is a bit more complex than just one string.
A guitar is going to have LOTS of information to describe it. Here’s an example of how you might create an Associative Array to describe the guitar. Then you can use that array in another array. You can compose these data structures in all of the ways. The trickiest part about learning to program – is realizing that after you learn the building blocks… the rest is really up to you.
Things can be incredibly confusing or so simple it hurts. It all depends on your choices. Some things are easy to read and pleasurable to use. Some things aren’t.
ALSO: did you notice that the first list item just prints as “Array?”
Well, if you follow the loop – the thing $guitar
reference is pointing to – is that $strat
things, which is an associative array and not just a string.
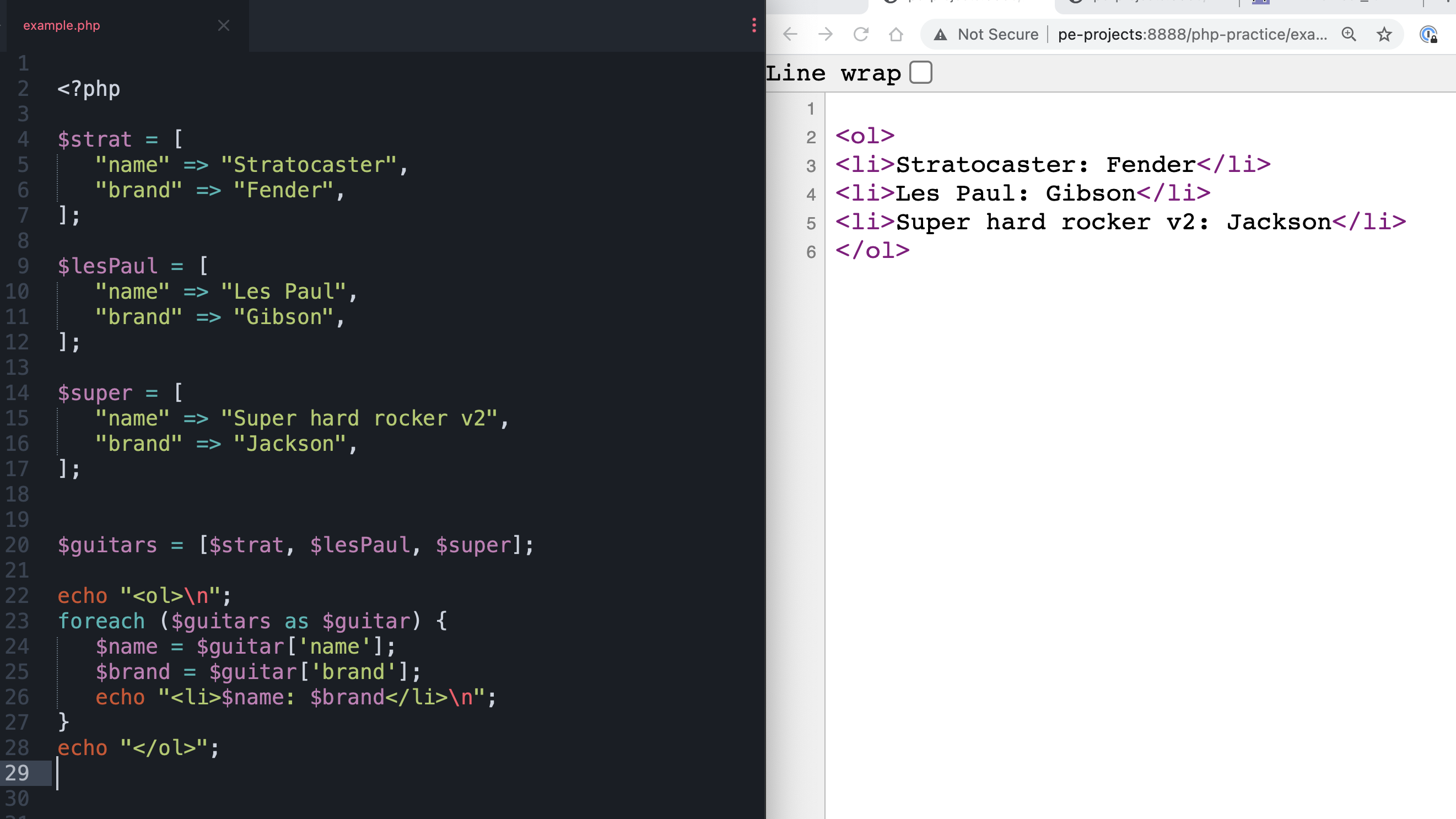
Here are a whole bunch of more complex guitars in an array.
Note the way we’re defining some concise new variables in that loop.
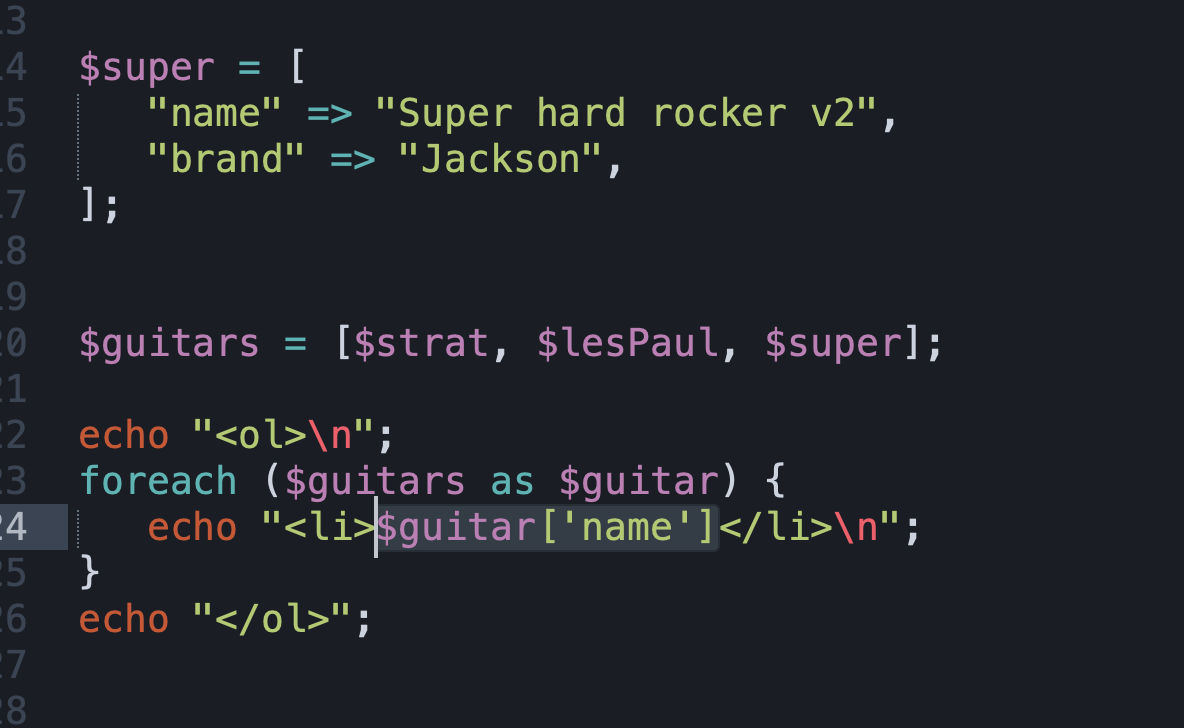
This sleek interpolation here – doesn’t put up with complex things like this. It’s a no-go, and that’s why we did what we did in the previous figure.
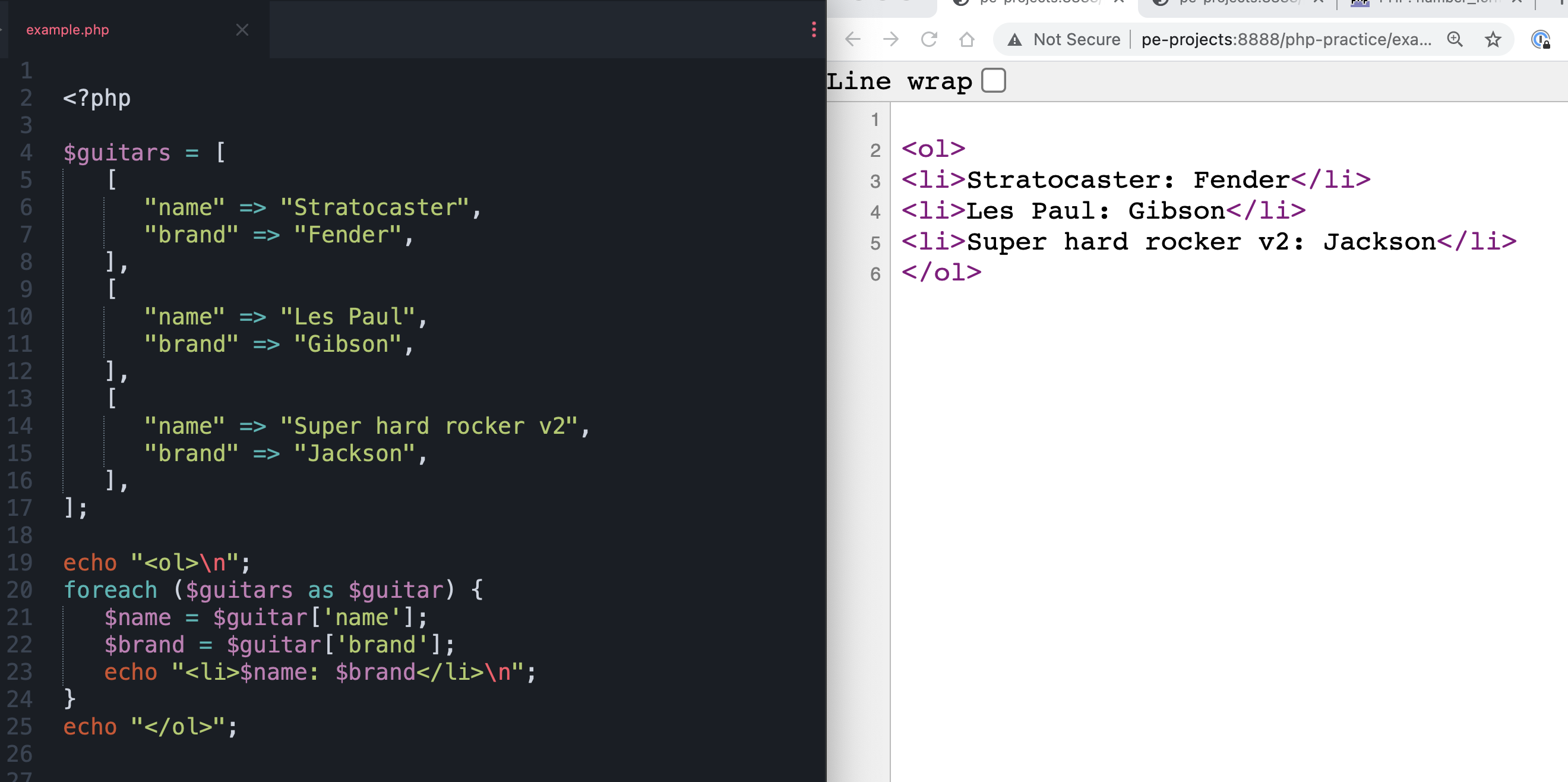
However it happens, the data ends up being in a larger data structure than just one guitar. You’ll likely have a huge dataset of nested arrays and objects and arrays. The guitars or products will likely be in an array of objects like this.
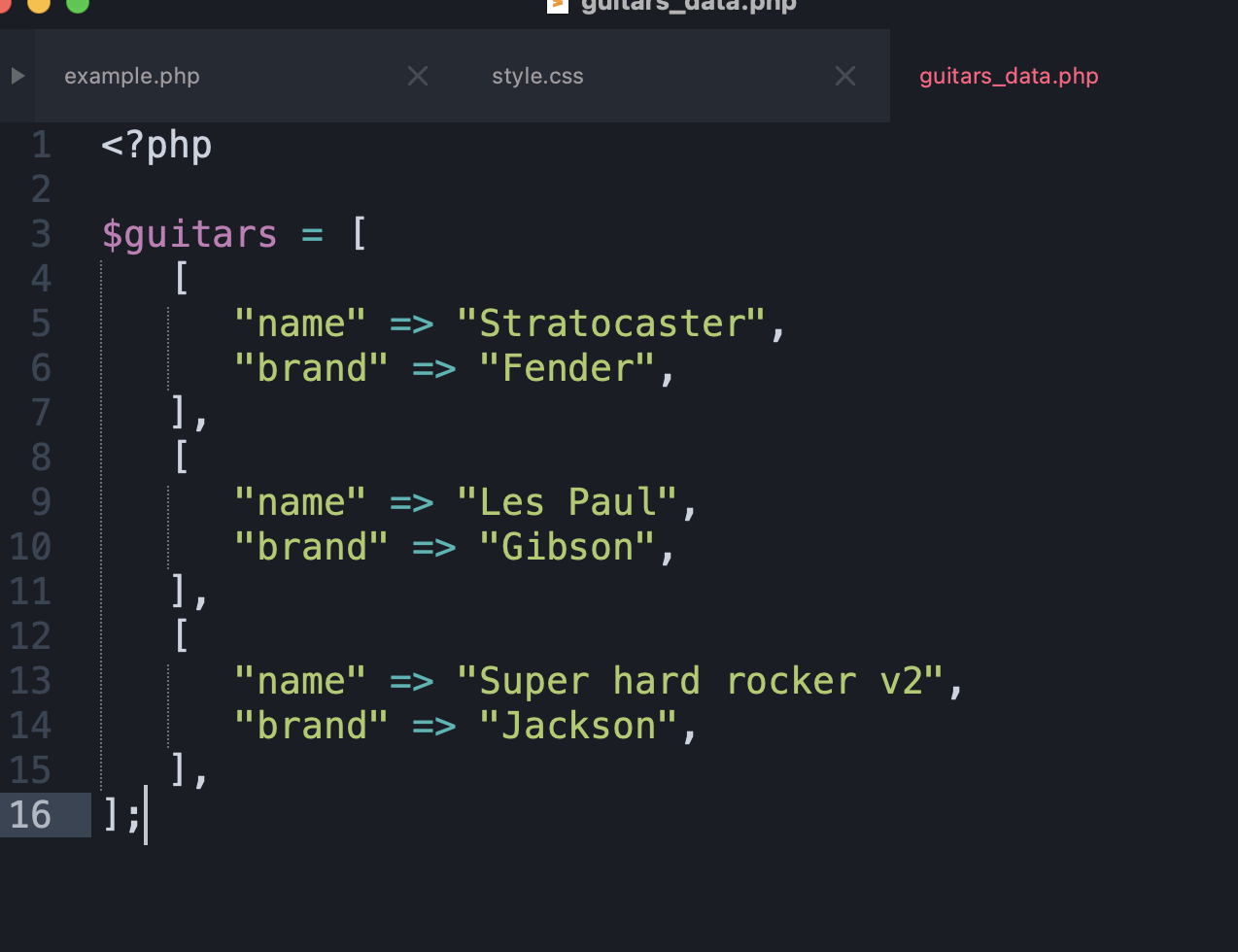
This is a teeny tiny little bit of data. You aren’t going to want all the code near your logic. You can move it to its own file. Sometimes you don’t even know what the data is. You just get it from some other website over the net.
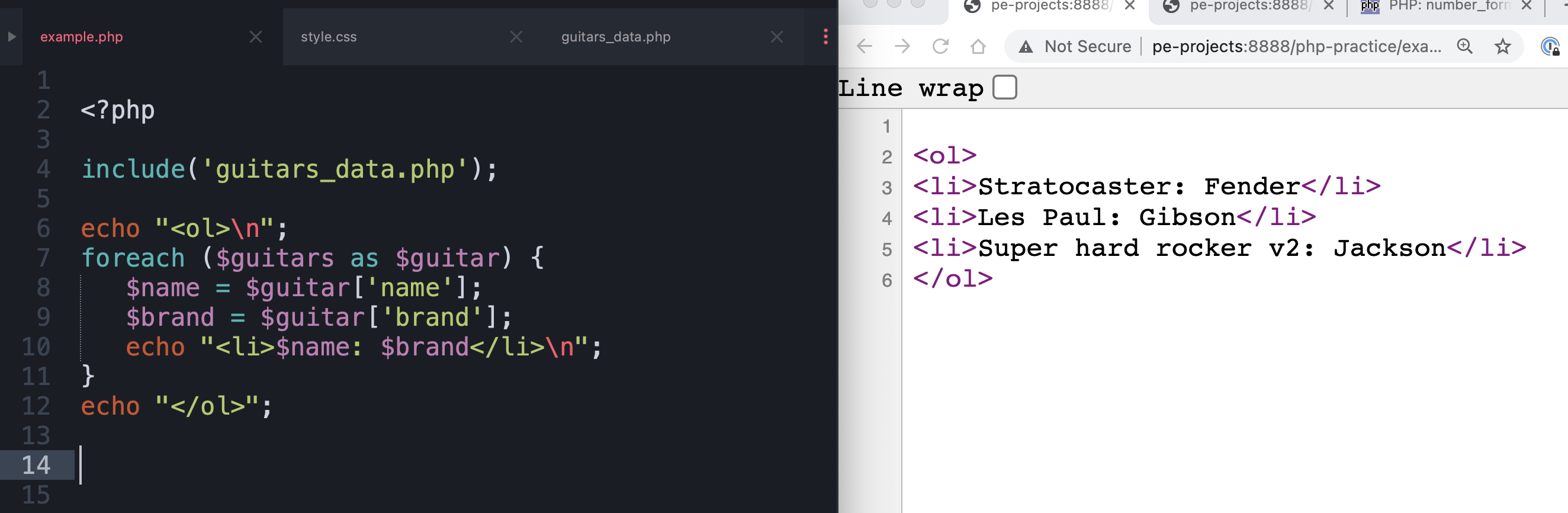
Here’s how you can include that other file in this one. You can’t see the list of guitars… but it’s getting glued in there.
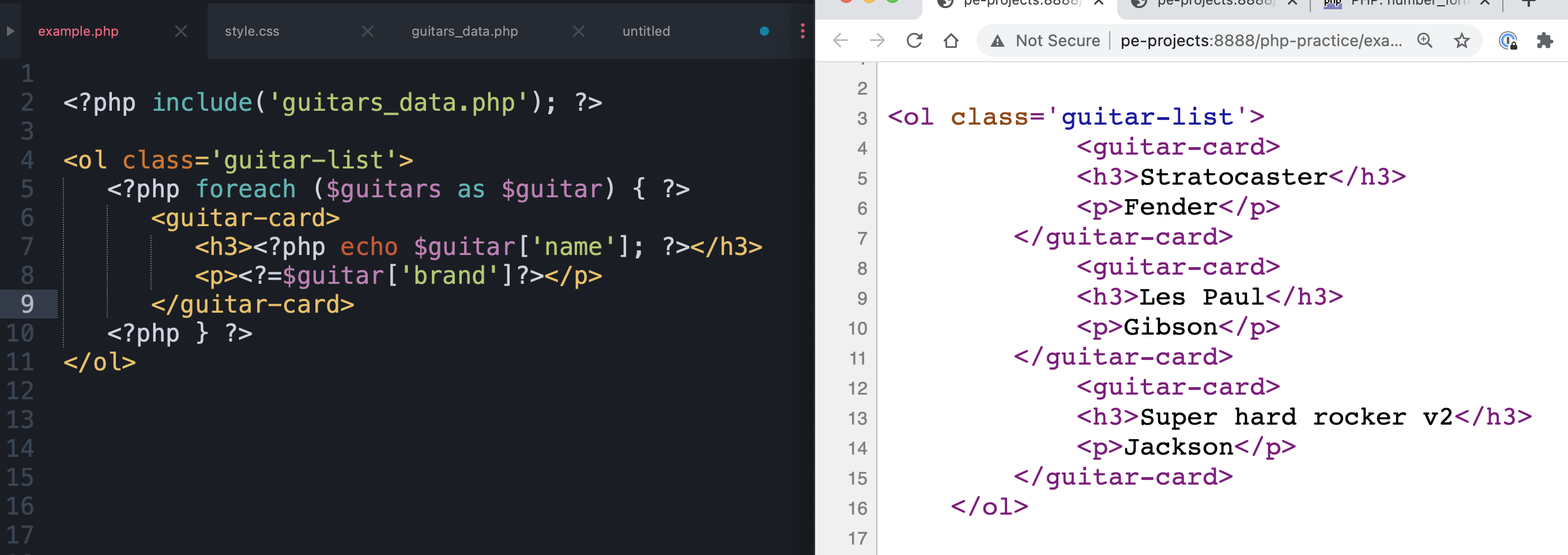
That figure before this one was all PHP style with echos and stuff.
You can mix PHP and HTML syntax ANY way you want – as long as you follow their syntax rules. You have to enclose PHP code in <?php
and ?>
– just like you have to enclose an HTML tag in <
and >
. It’s the rules. Follow the rules. Then you can do ANYTHING you can think of. Let that sink in. That’s where the art comes in. That’s where the creativity is really up to you. It’s freeing! But can certainly be confusing. “Wait, so – I can do anything?” (yes) “But how do I do it?”
Notice how we used that slick echo shorthand on line 8. We don’t dislike PHP… but we try to write our PHP to be as much like the other templating languages that we love as possible. This is more succinct.
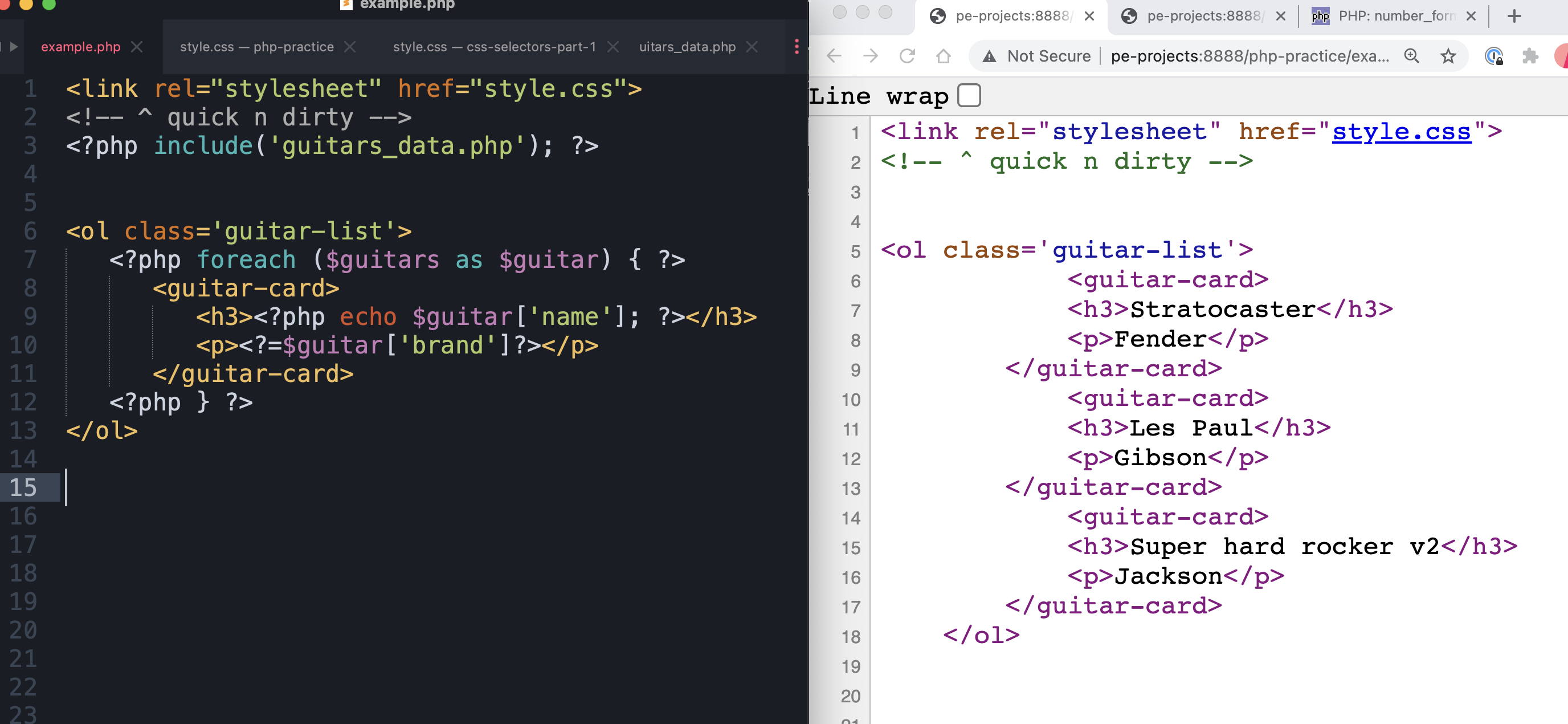
You might need some styling / but you may not want to spend the time to set up a whole site structure yet. This may be just a little prototype. You can use the link
element to connect a style sheet. It’s not really “officially” allowed outside of the head
, but the browsers will just fill in the gaps. It’s fine to play it fast and loose – when you’re playing it fast and loose.
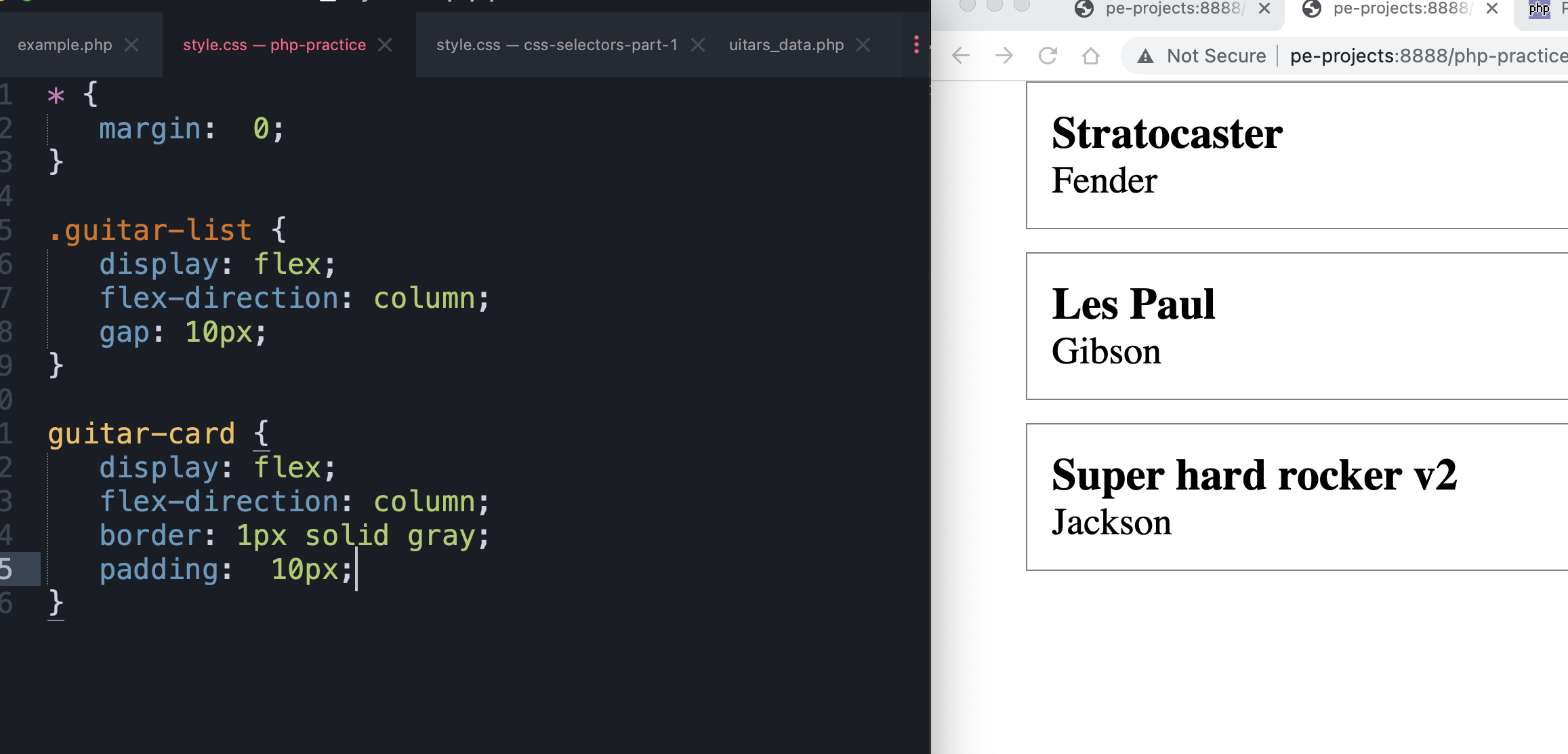
Here are some files.
See how the file got bigger and more complex, but then we broke it up into pieces?
OK. Take your time. Go over it again line by line. It’ll be fun!
Ask lots of questions.