Intro to JavaScript and the browser Console
Lesson Intro
You’ve got to start somewhere!
How about here.
The goals
-
Get a quick overview of what JavaScript is and why it exists
As you know, all the programming we’ve been doing requires a ‘server’ to run the code. This JS stuff can be run on the fly, right in the browser.
-
Learn what the console is and how to get there
Right in your browser is a “live” programming area. You can start your JS journey right there.
-
Go over the basic syntax
It’s mostly the same as PHP. Numbers, Strings, Arrays, Objects. How do you create them?
-
Run through a few challenges to get the hang of things
Writing JS is the lowest barrier of entry. You don’t even need a text editor, really.
Key
concept
Client
A “Client” is a funny name for an interface (hardware or software) that gives you access to a service provided by a “server” (another computer somewhere else). When you are using a “web application,” you are interacting with the client side of a client/server relationship.
WikipediaIn our case – the browser is the “client.” You’ve got your server-side code (scripts/programs) (the PHP) – and then you have this other client-side scripting called JavaScript. It’s going to run IN the browser itself. The browser has its own – what they call “run time.” It can execute your JavaScript right there – on the fly – in real-time.
Ain't nothing but a syntax thing
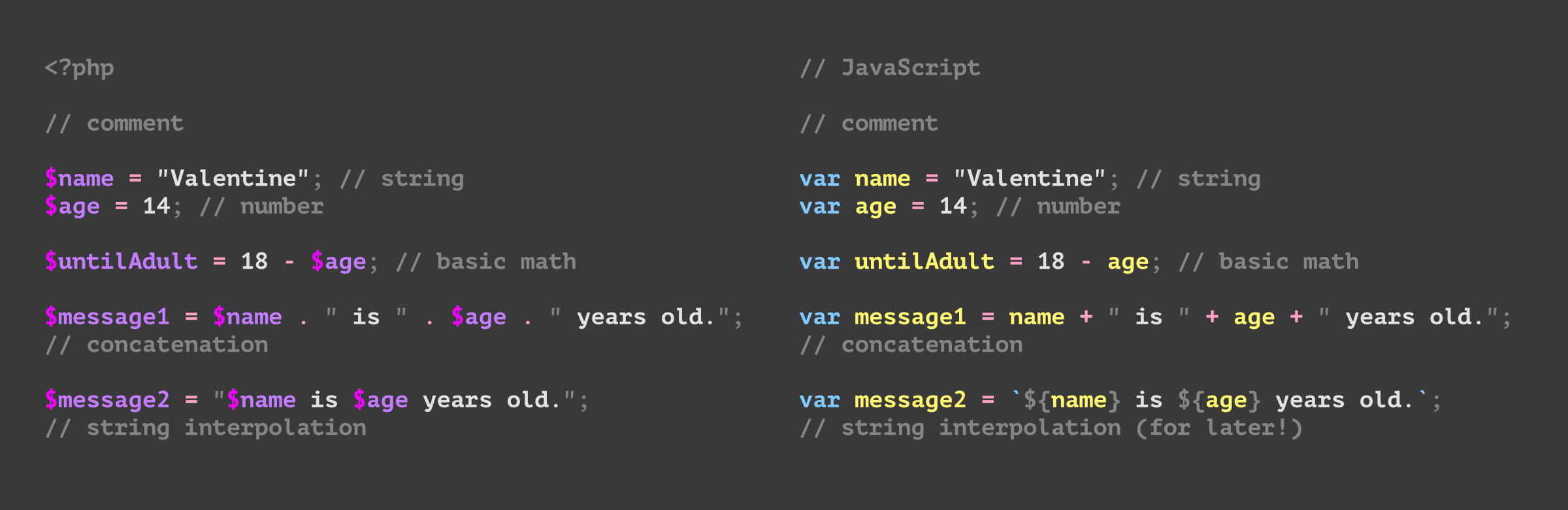
What percentage would you say these shared, syntax-wise?
The concepts sure are the same.
Things you can do
We can’t spend a whole day just practicing writing variables over and over again. That stuff doesn’t stick until you can do something meaningful. And it would be really boring. Plus, you already know a lot about PHP.
It’s not about learning “what” to type. It’s about learning “what you can do.”
-
Store something in memory
Just by typing something – you are putting it up there in the browser brain (memory). But that isn’t very helpful… unless you give it a name that you can refer to it as. You have to create a shared language between you and the computer.
-
Give something a name
By creating something in memory, defining a variable, and then pointing that variable to the thing in memory… you are giving it a name. That way you can use that name/reference to refer to that thing later. You and the computer can agree on what that word/idea means.
-
Do some basic math
You can add some things together… subtract them… multiply them etc. just the same as PHP. And of course – you can name numbers and their totals with variables.
-
Join words together
You can “concatenate” strings of characters together. The only difference is… that in PHP you use a
.
and in JavaScript, you use a+
You can also combine variables too, right? Because – they are often also just representing some strings of characters in memory.
-
Create lists of things
With Arrays, you can organize lists and then grab things out of them with bracket notation. This is the same as PHP.
-
Add things to existing lists
With the
array.push(item)
method, you can add items to an existing array. The item will get added to the end of the list. -
Describe complex concepts
With “Objects” – you can organize larger groups of data into sets of key:value pairs. They are like PHP associative arrays… but a more readable syntax with less typing! Hooray!
You can access the object’s data with
thing["key"]
bracket notation – but in JS, you can use an.
instead. It’s easier to type and read.thing.key
.. and you can add new values to an object with dot notation too!
thing.newKey = "Hey!";
Describing concepts
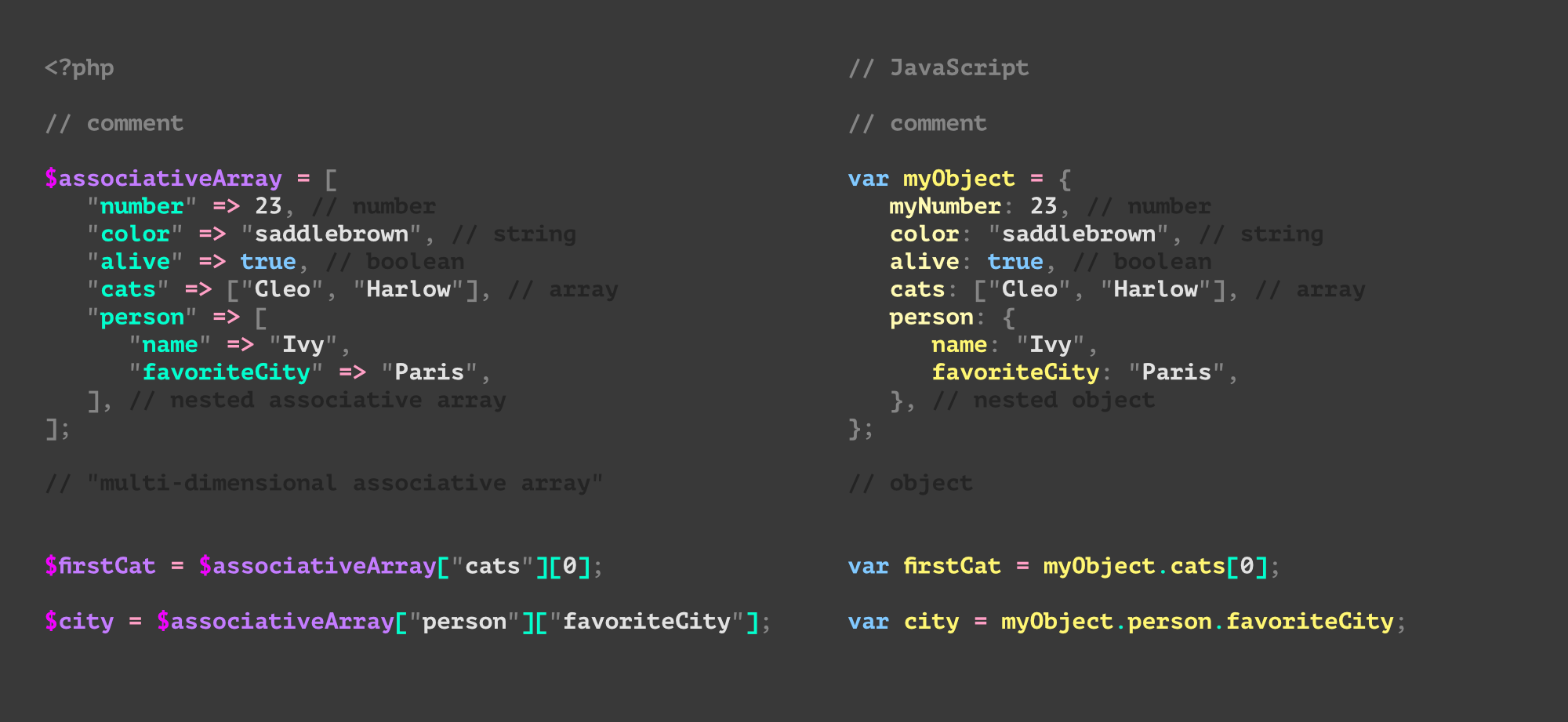
What percentage would you say these shared, syntax-wise?
The concepts sure are the same.
Which one do you like better?
Console?
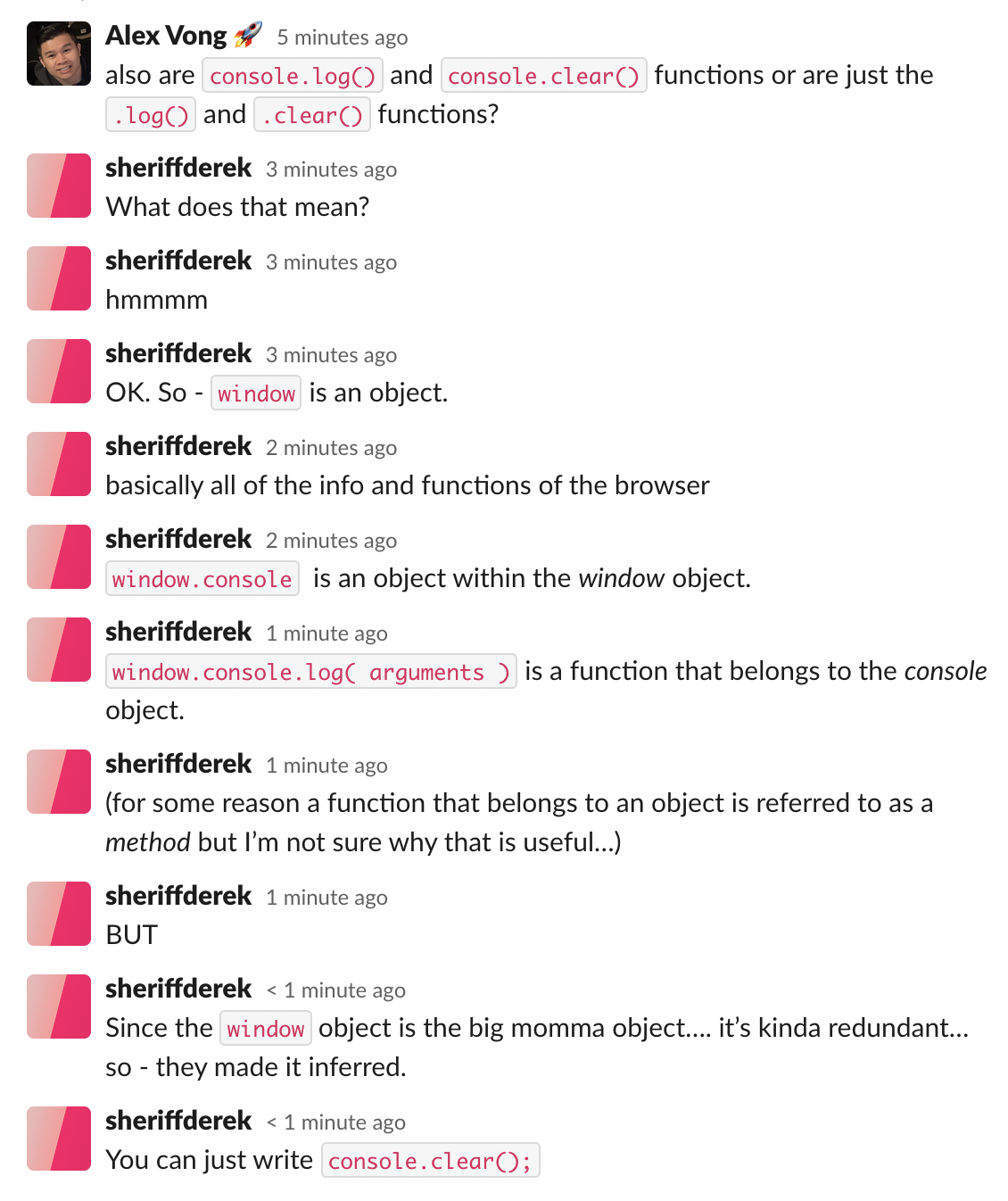
Had to think aloud for a minute…
log
and clear
are methods of the console
object. (which is not a JavaScript core language thing – it’s a part of the window
) (which is essentially the browser)
Newly introduced language features
-
JavaScript
Number
10
Doesn’t do much.
Imagine that you’ve put this in memory – but then immediately forgot where you put it and couldn’t find it again.
-
JavaScript
String
"A string of s0me characters."
Just like HTML and PHP. Choose double or single quotes. People probably used double because of apostrophes so they could say “Derek’s best friend Ivy” without confusing the computer. But as you’ll see, we have some new tools for this as well.
-
JavaScript
Declare variable (classic style)
var birthYear = 1982; var myWords = "Example string"; var hoursPerWeek = 7 * 8;
- Evaluate the expression on the right side of the assignment operator and place that value at some address in memory
- Create a unique reference(variable) name that you’d like to use to refer to that value (as made possible by the
var
keyword) (note camelCase) - Point the reference(variable) to that place in memory so that it can now act as a pointer to that stored value
Just like PHP but you need to use the
var
keyword and leave out the$
. -
JavaScript
Using variable
variableName
Just like PHP but with no
$
.If you had declared this variable, and pointed it to a value in memory…
(this seems redundant)
-
JavaScript
Math with JS
10 + 10; // 20 2 * 2; // 4
All the same as PHP.
+
.-
,%
,/
etc –(If you plan on using some advanced math, spend some time with the operator reference)
-
JavaScript
Statements in JS
2 + 2; var car = "Nissan Pathfinder.";
Just like PHP, statements should end with a semicolon.
However, JS won’t throw an error because it has very low expectations of you. Prove it wrong. Always use them while you are at PE. They are like the period at the end of a sentence.
Statements result in a value. Whether you create a reference to that value is up to you.
-
JavaScript
Concatenation
var message = "Hello, " + "Steve." + " How are you doing?"; var firstName = "Ivy"; var lastName = "Reynolds"; var fullName = firstName + lastName;
Just like PHP, but with a
+
instead of a.
-
JavaScript
Arrays in JS
var myDogs = ["Sparky", "Growly", "Softy"]; var myCats = [ "Skratchy", "Smelly", "Yucky", "Grumbler", ]; var secondCat = myCats[1]; // "Smelly"
Same as PHP.
-
JavaScript
Array.push()
myCats.push("Cranky"); // ["Cranky"] myCats.push("Whiskers", "Claw"); // ["Cranky", "Whiskers", "Claw"]
Instead of
array_push($array, $itemToAppend)
, you chain on to the array itself with this method.You can add additional items by separating them with commas.
-
JavaScript
Objects
var person = { name: "Valentine", age: 15, alive: true, thingsSheLikes: undefined, } var age = person['age']; var name = person.name; // // a regular array is really just a convenience var dogs = ["Sparky", "Growly", "Softy"]; // 0 1 2 // is really an object behind the scenes var dogs = { 0: "Sparky", 1: "Growly", 2: "Softy", }; var found = dogs[2]; // "Softy"
Mostly the same as an associative array in PHP, but no quotes necessary on the keys, colons instead of the
=>
, and there’s this “dot notation.”You’ll almost always use the
person.name
style of accessing properties, but theperson["name"]
“square bracket notation” is super useful too – as you’ll see later. -
JavaScript
Functions
function functionName() { // run the program described in this codeblock console.log("I'm a program"); } functionName(); // logs "I'm a program" function alertName(parameter) { alert(parameter); } alertName("Anna Lena"); // alerts "Anna Lena" function doubleNumber(numberInput) { return numberInput * 2; } console.log( doubleNumber(4) ); // 8 function doThings(parameter, greeting) { // do things with parameters console.log(`${greeting}, my name is ${parameter}`); // or not } doThings("Marco", "Hola"); // logs "Hola, my name is Marco." var cat = { name: "Hairy", age: 7, skreetch: function(sound) { alert(sound + "!!!!"); }, }; cat.skreetch("wraahhwwwwrrrrrr"); // alerts "wraahhwwwwrrrrrr!!!!"
These are the same as in PHP, but no
$
on the variables.Also, you can use functions them as any value like in this object example.
We didn’t talk about true objects in PHP (unless you wanted to), so – this will be the first time you’ve seen a “method” which is a function that belongs to an Object. As you can see, it’s a property – so you access it the same way as any property but you call it with the
()
.
Exercises
-
Watch the video and follow along
Practice with the JavaScript syntax. Make sure you try all of the things discussed.
-
Practice with some of your own ideas
You’re going to have to just try a LOT of things for it to click. Have at it!
-
PHP and WordPress aren't over
Keep on truckin. We’re going to need that CMS setup for next week – so we can use it with JavaScript.
More things!
(If you’re interested in this type of stuff)
Lex interviews Brendan: https://www.youtube.com/watch?v=krB0enBeSiE
TONS of great history and inside information.
Also: this: https://codepen.io/sheriffderek/pen/EyQVqv?editors=1000