Why we chose to teach Vue instead of React
Introduction
Why don’t we teach React.js in the core curriculum at PE?
How about a better question: How can we teach someone everything they need to know – while simultaneously allowing them to feel confident and productive – the whole time? (even though there is so much info getting added in every day?)
We’ll use whatever tools help us accomplish that.
Here's a chart we found
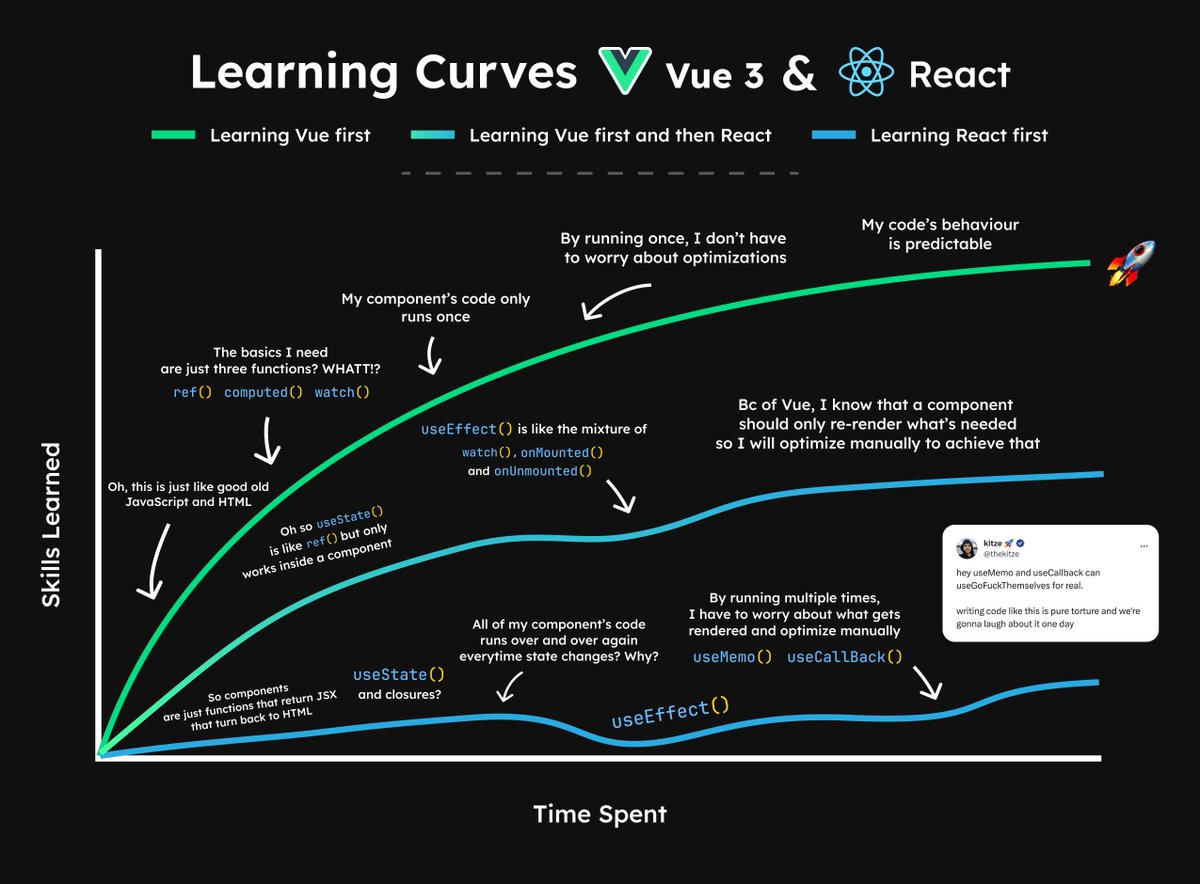
@icarusgkx drew this up. It probably won’t make any sense right now. But it’s saying that if you learn Vue.js first (as opposed to learning React.js first)… then things will be much less confusing. Once you have a handle on that, then the more complex parts of React.js will be easier to understand and break down with the foundation you’ve already built.
We care about the students being productive enough that they can actually make things. Vue makes that happen. And then – when/if you go to learn React, you’ll have confidence in the larger system, and it’ll be much easier to translate. By teaching you ‘how to build web applications’ – we are teaching you React. You just don’t know it yet.
But there are so many things you should learn (in detail) before you consider any of these frameworks.
Still writing this out!
Just having some morning thoughts – and getting them out there / to flesh out later – –
- NOTEs…
- HTML (gets you data structures and content strategy) (accessibility) (also has OOP if you can handle seeing it that way early)
- CSS (gets you object syntax and object oriented concept basics)
- PHP (gets you programming logic and serverside scripting)
- JS (gets you more programming logic and the web api and clientside scripting and async and integration with 3rd party code)
- Node (JS on the server / and explains how and why the build tools are needed) (building an API) (you could skip the PHP and do this – but there’s WAY more overhead and the timing isn’t good / and it’s lazy)
- Vue (puts that all together and solves most of the problems you found here ^)
- Nuxt (integrates the client and server into one repo)
- then you can quickly pick up React / if it’s still a thing by then –
-
How the internet works
Kinda important.
-
HTML
-
CSS
-
PHP
…
also, add a note to this one… if you learn a little more about this at the time… then you’ll already basically know Typescript when you get there 😉
-
CMS
Make your own / then you can see just how things like WordPress work – and how the CMS stuff you’ll make later with JS will work.
-
JS
Cause we need to change the DOM
-
How to integrate other people's code
using things like flickity – or lodash
-
Node
Module systems and libraries
-
-
Building a server and API
-
Vue
-
Database and CMS as a service
-
Nuxt
How coding bootcamps do it
Let’s just be honest
-
JS, terminal, Git, HTML, CSS
They’ll ask you to learn all this before the course starts – or they’ll dedicate a shitty week or two to blasting out crappy HTML and broken layouts. Maybe that’s all we can expect from people, though. It usually amounts to knowing as much HTML and CSS as high school student in 1999 could learn during their lunch hour in the computer lab.
-
React
Some schools will spend a lot of time on foundational JS stuff. It’s true. But also, they don’t teach you how to use it – and then just jump straight into React and get you cobbling together libraries and learning how to cut every corner you can. Look at any boot camp student’s portfolio or github code. The proof is there.
So, what you are learning is “React” – which is an abstraction layer. So, you’re learning a specific interaction layer and a specific API. That API will change. That API will eventually go away.
People survive. It’ll be OK. But – it’s not a very smart way to roll out all of these concepts. We can do better. It’s actually easier to just do it well.
Diversity
These examples are just the templating side of things. We could talk about handlebars, or string literals, or liquid templates, or ejs. But we’re not training one type of person to be a general full-stack coder dude. That’s not the only job. In fact, it’s just a small slice of the pie. And at real jobs, you aren’t flailing around pretending you’re a one-man coding machine.
At real jobs, normal everyday developers need to be able work within your templates. People of all skill levels and specialties need to be able to contribute.
Vue has the most shareable API. That’s just a fact. You can learn it progressively with much fewer syntax barriers. It’s better for everyone – and especially for when you’re learning.
Evolution
<ul class='product-list' role="list">
<li class='product'>
<article class='product-card'>
<h1 class='title'>Product name #1</h1>
<picture>
<img src='...' alt='' />
</picture>
<a class='link' href='#'>
Read more
<span class='screen-reader-only'>about Product name #1</span>
</a>
</article>
</li>
<li class='product'>
<article class='product-card'>
<h1 class='title'>Product name #1</h1>
<picture>
<img src='...' alt='' />
</picture>
<a class='link' href='#'>
Read more
<span class='screen-reader-only'>about Product name #1</span>
</a>
</article>
</li>
<li class='product'>
<article class='product-card'>
<h1 class='title'>Product name #1</h1>
<picture>
<img src='...' alt='' />
</picture>
<a class='link' href='#'>
Read more
<span class='screen-reader-only'>about Product name #1</span>
</a>
</article>
</li>
</ul>
This might look like gibberish to you. But what we’d like you to take away – is that there’s a lot to type! And a lot of places you could mess things up – and a lot of repeated code. And also, try and imagine that there are 100 products coming from some database somewhere. You can’t type them all out by hand. What if the prices get changed? Or things sell out?
<?php
// what a product's data might look like
$products = [
[
"title" => "Pink football",
"image" => "...",
"image_alt" => "..."
]
...etc
];
?>
<!-- $products comes from the database -->
<ul class='product-list' role="list">
<?php foreach ($products as $product) { ?>
<li class='product'>
<article class='product-card'>
<h1 class='title'><?=$product['title']?></h1>
<picture>
<img src='<?=$product["image"]?>' alt='<?=$product["image_alt"]?>' />
</picture>
<a class='link' href='#'>
Read more
<span class='screen-reader-only'>about <?=$product['title']?></span>
</a>
</article>
</li>
<?php } ?>
</ul>
You can use PHP with very little setup and very little understanding of programming. It generates the HTML page you see up above. That’s all. It generates an HTML page. There are no interactive elements. That’s really important to understand early. Later, you might spend a lot of time trying to get you’re JavaScript app to be server-side rendered. This way, you’ll actually know what that means – and have had time exploring the strange side of that world. If you can’t get comfortable with how a server programmatically builds HTML pages with data and your simple instructions – then you really shouldn’t move on.
// there would be a bunch of JavaScript learning in here... and it'll get pretty ugly at times...
// and it's not part of out point here - but you'd learn a lot of behind the scenes things before you end up using Vue.js
<script>
// what a product's data might look like
var products = [
{
title: "Pink football",
image: "...",
image_alt: "..."
}
...etc
];
// but would come from the database
</script>
<ul class='product-list' role="list">
<li class='product' v-for='product in products'>
<article class='product-card'>
<h1 class='title'>{{product.title}}</h1>
<picture>
<img :src='product.image' :alt='product.image_alt' />
</picture>
<a class='link' href='#'>
Read more
<span class='screen-reader-only'>about {{product.title}}</span>
</a>
</article>
</li>
</ul>
With Vue, the templating is HTML with just a little magic.
function ProductList({ products }) {
return (
<div>
<ul className='product-list' role="list">
{products.map((product) => (
<li className='product'>
<article className='product-card'>
<h1 className='title'>{product.title}</h1>
<picture>
<img src={product.image} alt={product.image_alt} />
</picture>
<a className='link' href='#'>
Read more
<span className='screen-reader-only'>about {product.title}</span>
</a>
</article>
</li>
))}
</ul>
</div>
);
}
We might want to make some notes about JSX here? – or compare Svelt… but maybe not — (this example is too simple to make the point)
Really.. the point is that by learning PHP and JS and then Vue (well) – you end up learning all the frameworks subconsciously. If you learn React first – as your primary entry point / you’re going to be stunted in many ways –