The Document Object Model
Lesson Intro
The browser gets the HTML page. It builds out a wild tree of information about it – and makes that information available to you. Then you can interact with it and change it – right in the browser.
Thanks, browser! That was so cool of you!
The goals
-
Get down with the DOM
Do that thing. Spend some time digging in and molding your mind to the new tree of nodes.
Key
concept
DOM
When you make an HTTP request (visit URL or click a link) the browser will download a copy of that file. It will then read it over and construct its own version of that HTML page. Think of it as a JavaScript Object. A bunch of little collections of key:value pairs that describe the page structure and content – so that the browser can render the page – and also so that YOU can interact with it.
“The Document Object Model” is quite a mouthful, so – most people just call it “The DOM” – or when referring to parts of it, “DOM.”
What is the "DOM"
-
Ire has a nice writeup here
https://bitsofco.de/what-exactly-is-the-dom
Also – consider diving into her article on “The critical rendering path” https://bitsofco.de/understanding-the-critical-rendering-path (just for fun)
-
JavaScript.info's visual example
This has some nice interactive representations: https://javascript.info/dom-nodes#an-example-of-the-dom
-
How the browser renders a page
https://medium.com/jspoint/how-the-browser-renders-a-web-page-dom-cssom-and-rendering-df10531c9969
-
W3C
W3C Level 1 Document Object Model Specification.
-
Wikipedia
-
But don't get stuck too deep in that stuff...
Find something that helps you understand enough to start interacting with the DOM. You want to be a designer – and not a DOM expert right now.
People complain about HTML. They complain about CSS. They complain about JavaScript. But – sometimes people say… that most of the trouble with those things is actually because the DOM maybe sorta could have been better…
But we’re just going to learn to do the work. There are enough excuses out there without adding things that are out of your control.
Shortcuts
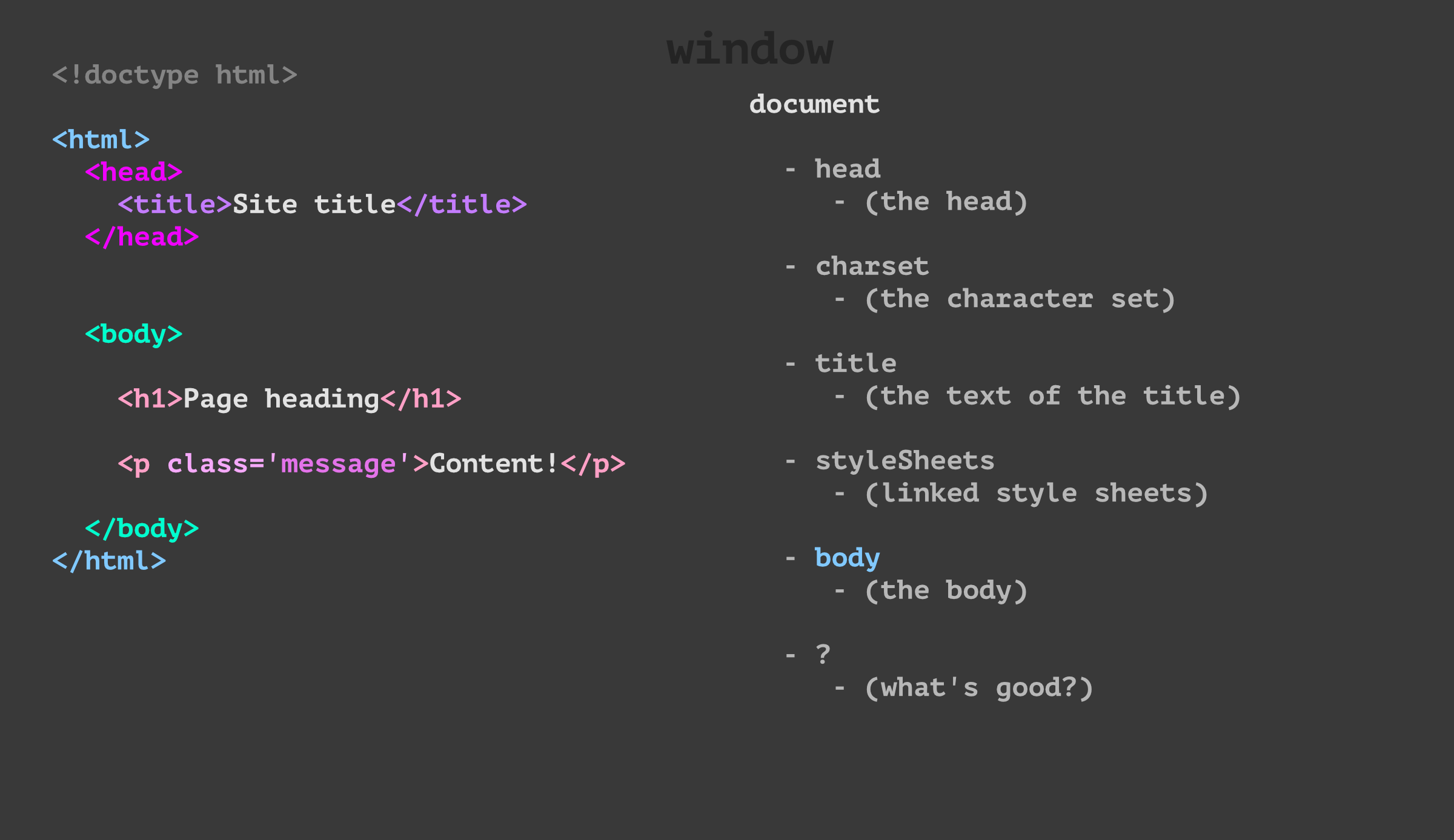
We don’t think anyone explains this very clearly. You just “do stuff” and then your brain fills in the gaps with misc. justifications. (We don’t think that is a good thing!!!)
This is how we think it’s best to break it down:
There are some ‘shortcuts.’
document.certainThings
– just goes straight there. Why? Because.
There are a few useful ones, but – we consider these shortcuts to be the flukes.
Also, note that above the document – there’s the window object. It has the location, document, and history objects. (You can think about that in the future if it ever matters to your work)
DOM nodes
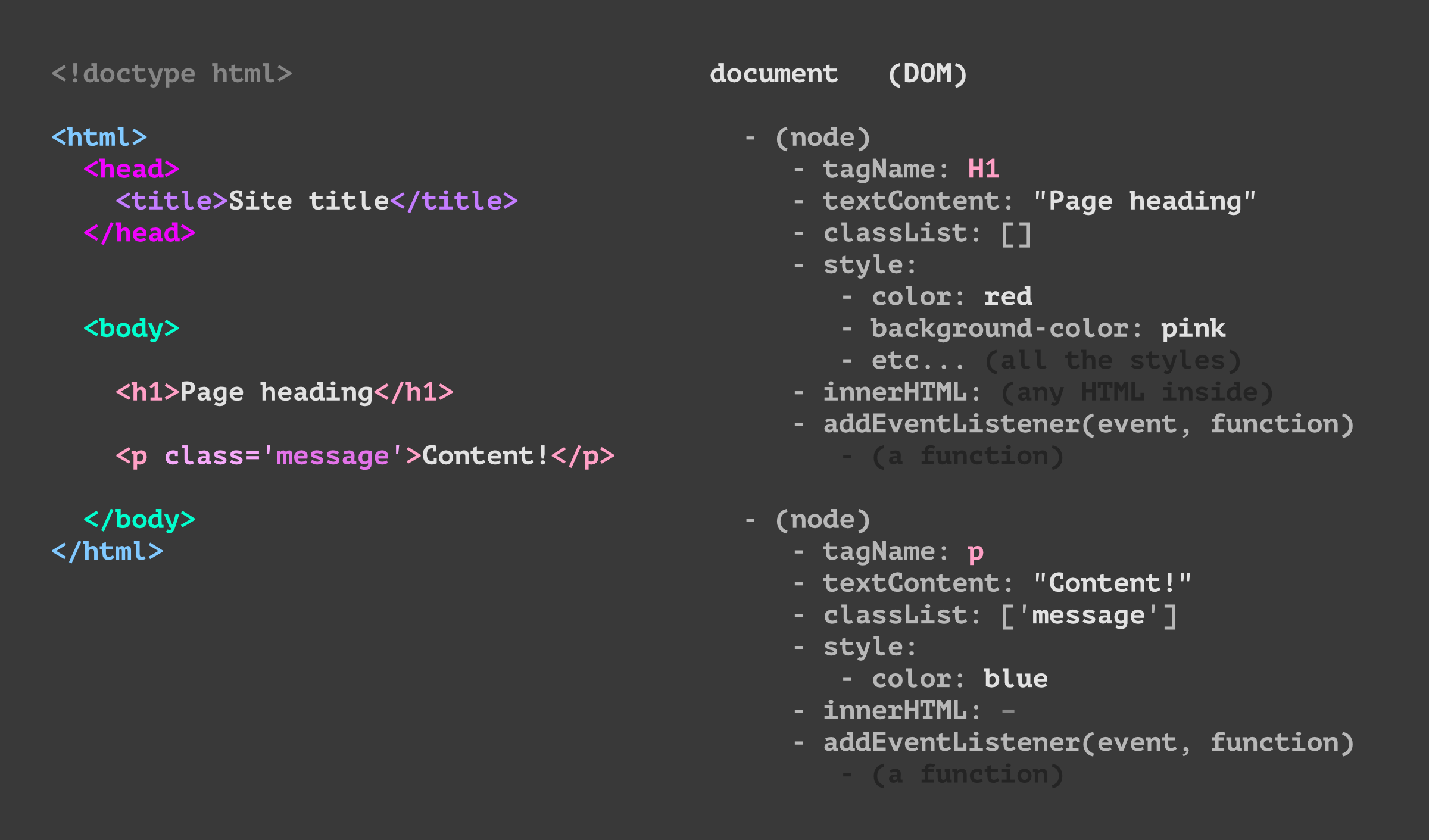
Most of what you’ll be doing is a lot more like this.
You’ll need to query the DOM for various elements. You can get properties – and set properties, which is a concept that blends over to most of the languages.
var heading = document.querySelector('h1');
heading.textContent;
(returns/gets the textContent property value)
heading.textContent = "New heading now!";
(sets the textContent property value)
It’s actually very elegant. (although unnecessarily mysterious)
(this diagram just shows a few of the ones we use most — but there are WAY more) (we’ll tell you about them in order of necessity)
Questions
Everyone says they want to learn JavaScript. Why?
-
What are some “JavaScripty” things that people want to learn?
-
Why would we need JavaScript?
-
Where have you seen it used?
-
What are the pros and cons?
Exercises
-
Obviously - watch the video...
Pause it and try out the things.
-
Mess up someones page
Find a website and use the console to change a bunch of stuff on their page! You can insert things into the page – and use the style property of elements to change the CSS. Hint, you can use
.appendChild()
on any element. -
Create a page with only JavaScript in the console
Practice using the
document.createElement(name)
to create and build out elements. Then put them all on the page. Take a screenshot of the page – and share it with us. How complex can you get – in 1 hour? Have FUN!
Newly introduced language features
-
JavaScript
Comments
// single line comment var age = 22; // or here! /* multi line comment */ var favoriteCity = "Paris"; /* as long as there's a start and and end (just like CSS comments) */ var timeOfDeath = undefined; /* the space doesn't matter */ /* but you can still make things look nice, can't you? */
Comments. You probably aren’t going to be writing them in the console, but when you get to the text editor they’ll be very important. Use them.
-
JavaScript
.querySelector()
// document.querySelector( CSS Selector ); var myHeader = document.querySelector(".site-header"); var myMenu = myHeader.querySelector(".site-menu");
Returns the first
Element
within the document/element that matches the specified selector, or group of selectors.If nothing in that scope matches, then it will return
null
. -
JavaScript
createElement()
// document.createElement(desiredElementName); var myHeader = document.createElement('header'); myHeader.classList.add('site-header'); document.body.appendChild(myHeader);
This creates an official node.