User input with browser dialog boxes
Lesson Intro
Forms are a bit easier to build in JS than in PHP… but they still require more working knowledge than you have. Until then, we can use these browser-based dialog box things.
It’s probably the fastest way to get user input – out of all of the programming languages.
The goals
-
Master alert, confirm, and prompt
A few solid sittings should burn these into your brain.
-
Start thinking more about functions
What are they. What are they good for? When can you use them?
-
Get a little more practice with control flow
You should have this if/else stuff down pretty good by now, but – these dialogs will reveal many uses for control flow.
Key
concept
Dialog box
These little boxes are a way to communicate with the user. They may or may not block the content behind them, but as the name suggests they are to interact and have a dialog with you. The computer might alert you to a change, warn you of the outcome of your choice, or ask you for some input. macOS uses them heavily and so do web browsers, but any web application can employ their own version of the concept.
WikipediaIn our case, this is a browser-specific dialog box. But surely you’ve seen your MacOS dialogs that prompt you for passwords and tell you to brush your teeth and do your homework.
It’s a very general term. It’s a “conversation.” You are having a dialog / in a box!
Recap
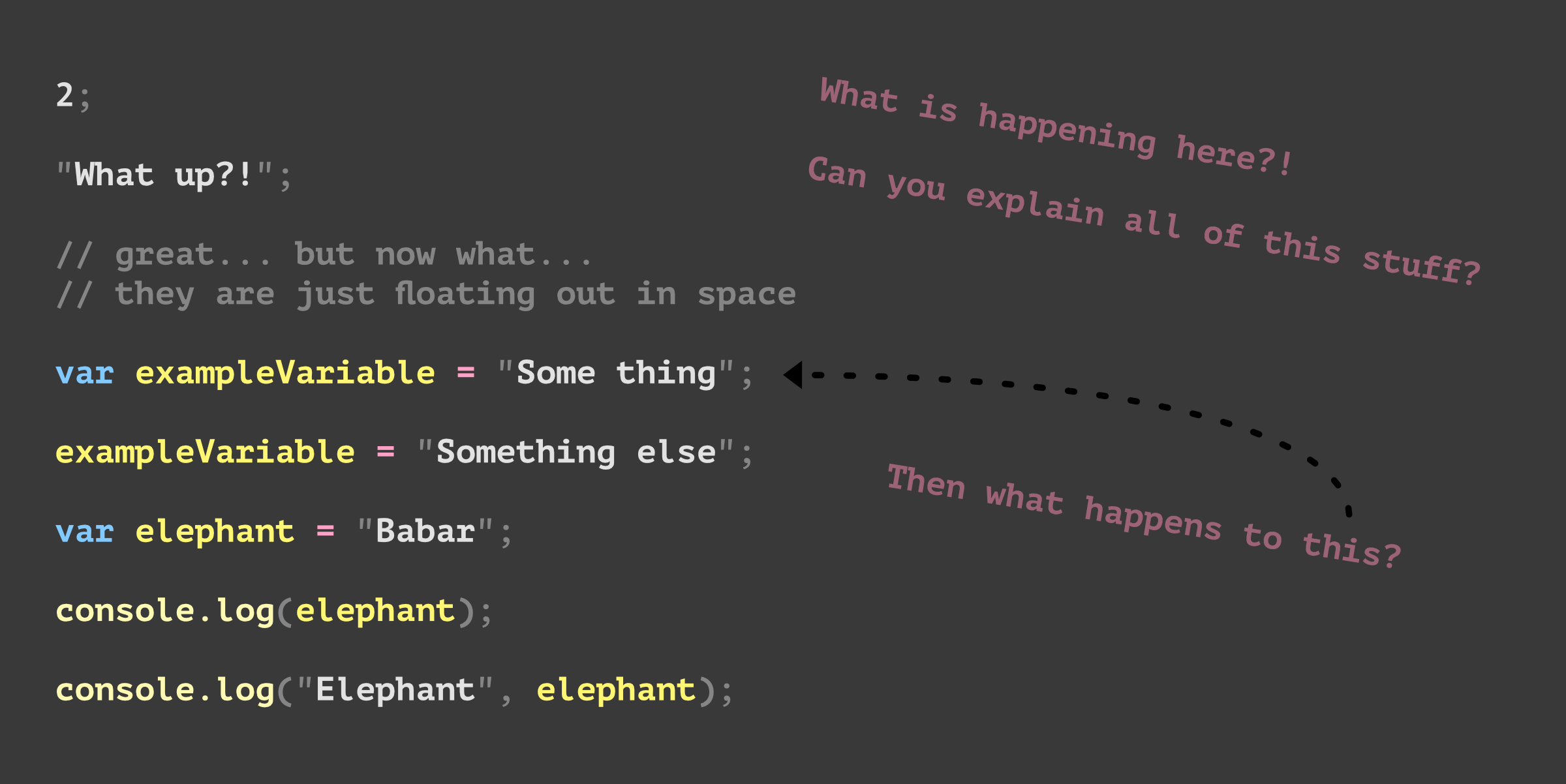
Can you explain everything that is happening here in detail? If not – then work it out: today!
Top down
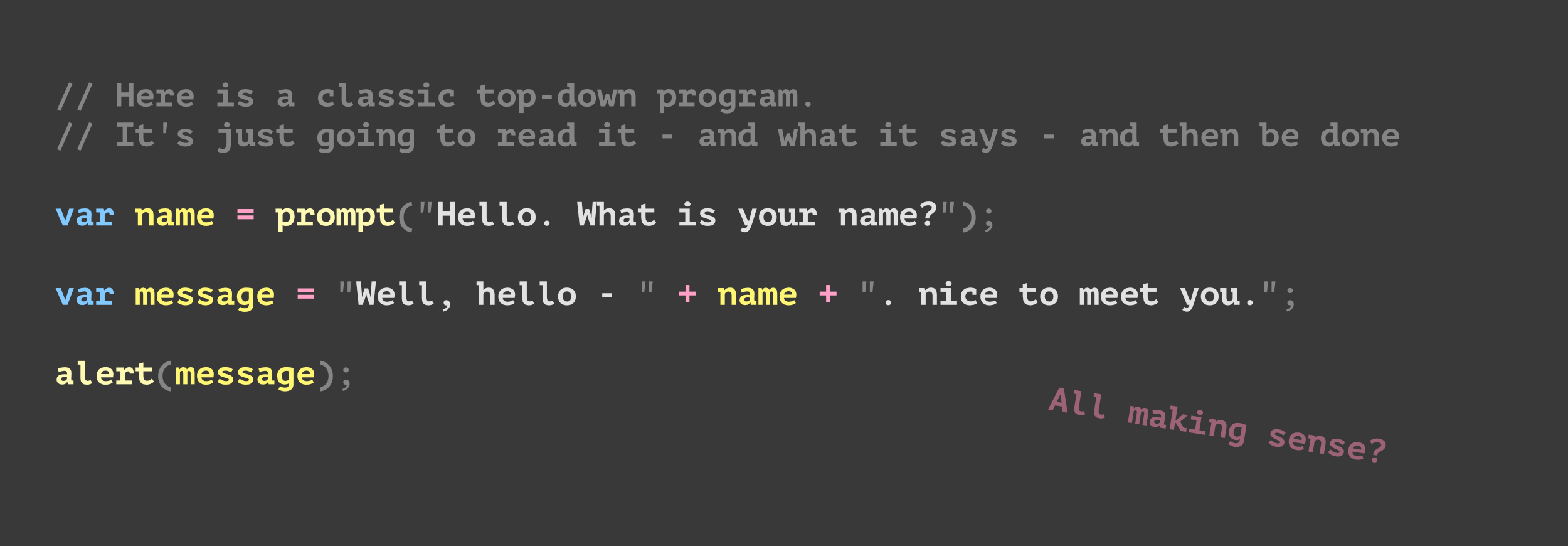
These directions are just floating out there in the global space. They will be read top-down – just like PHP (for the most part).
An encapsulated program
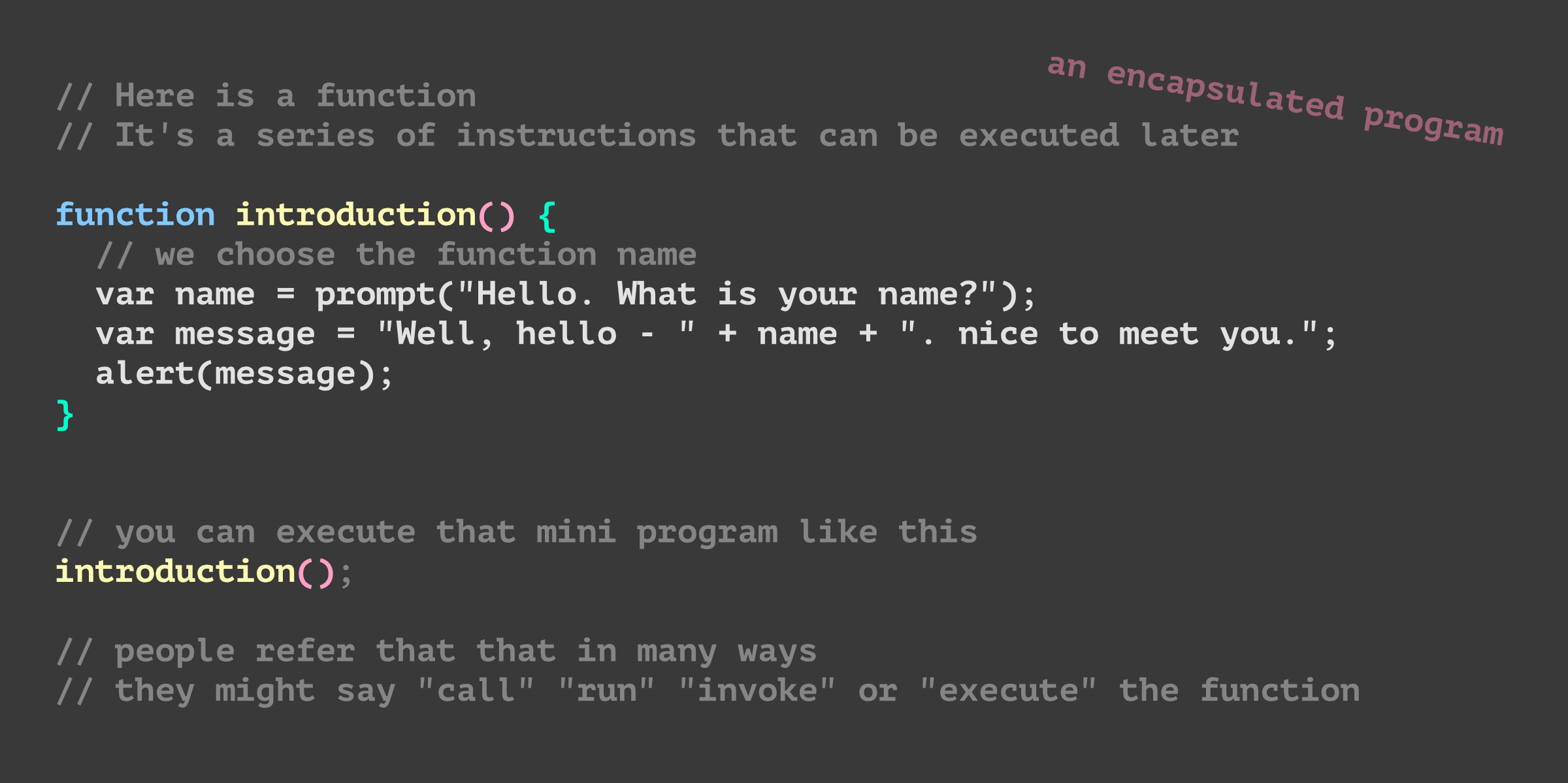
Functions can be defined (designed) – and then used whenever and wherever – and as many times as you want / at any time – while the page is loaded.
Sometimes you use them to just run some code. They can almost be used like a partial. Just a set of instructions.
Return values
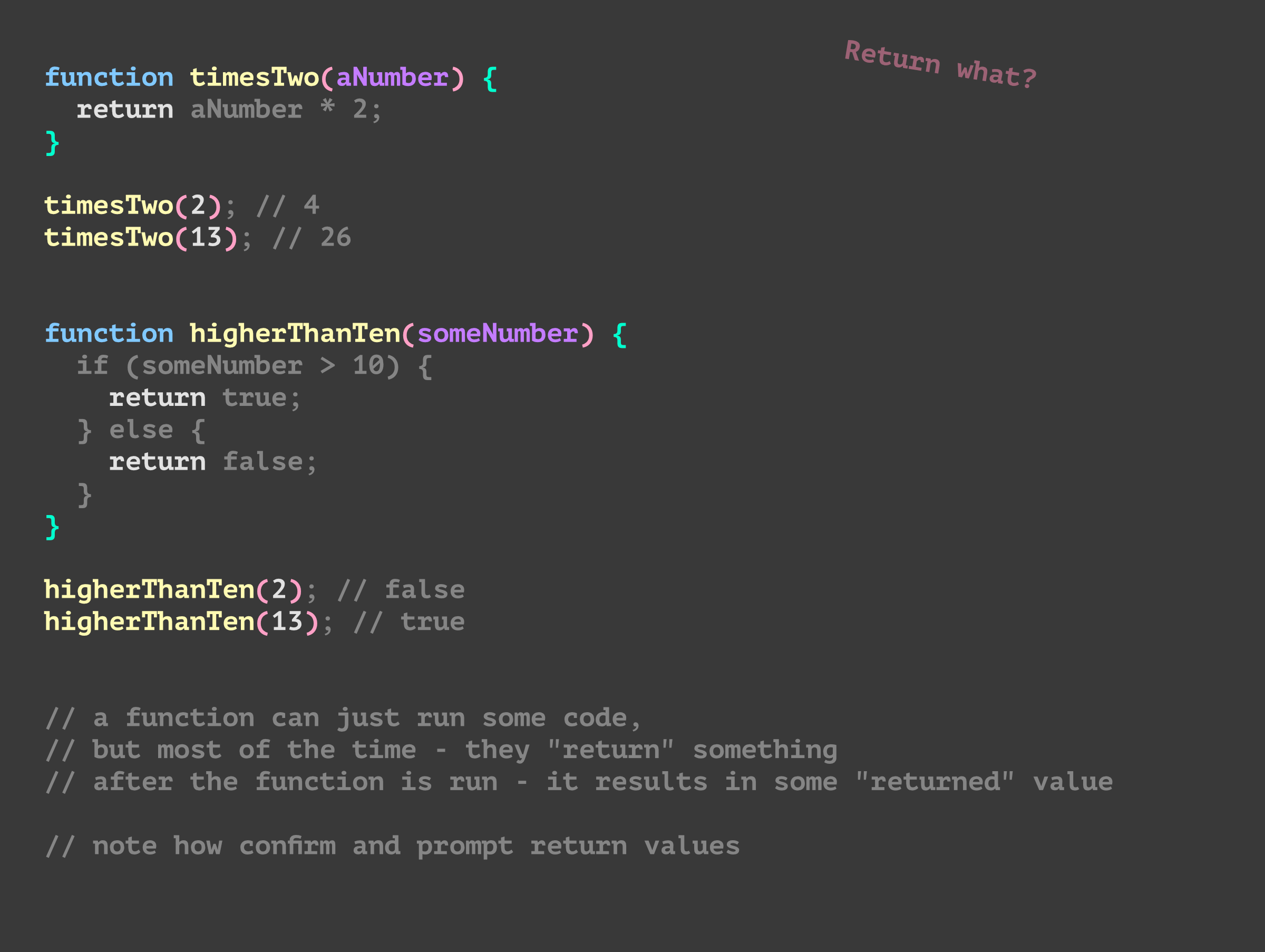
Dialogs
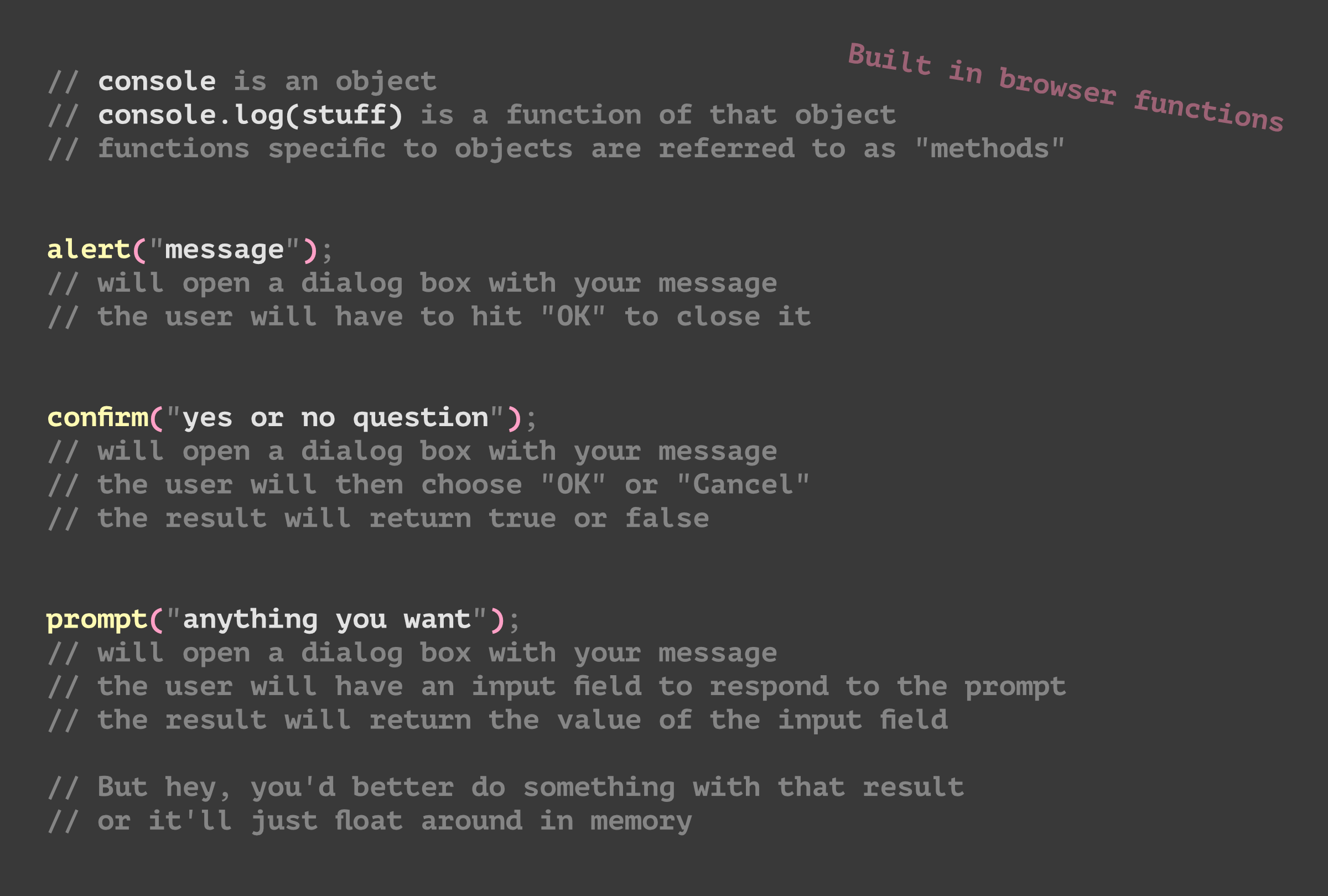
Does this make sense? If not — you haven’t practiced enough.
Browser dialogs
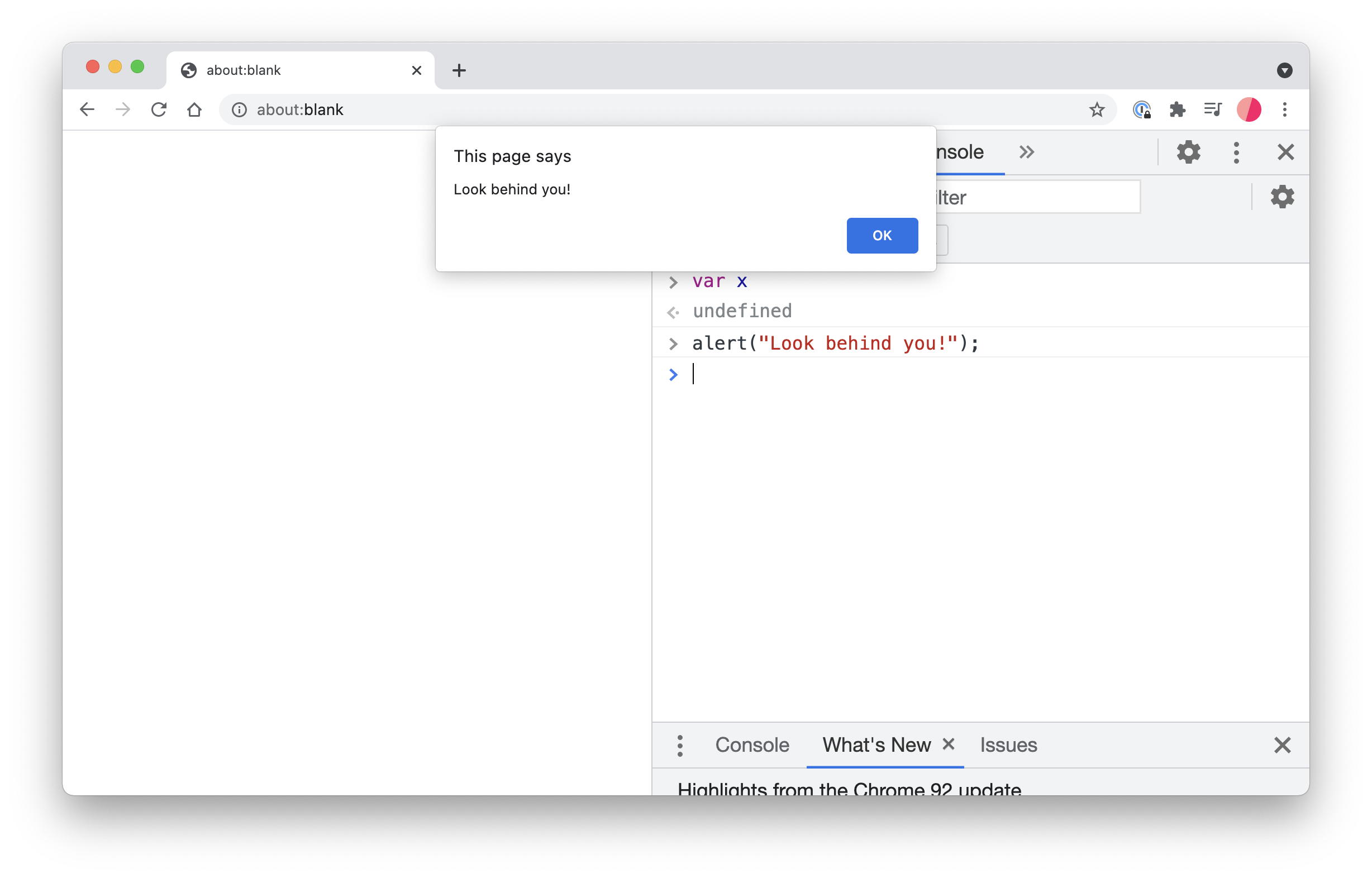
They look a little different in each browser.
JavaScript
alert()
// window.alert()
alert("Message");
Creates a modal dialog box to present the user with a message.
This is specific to the browser.
Alert
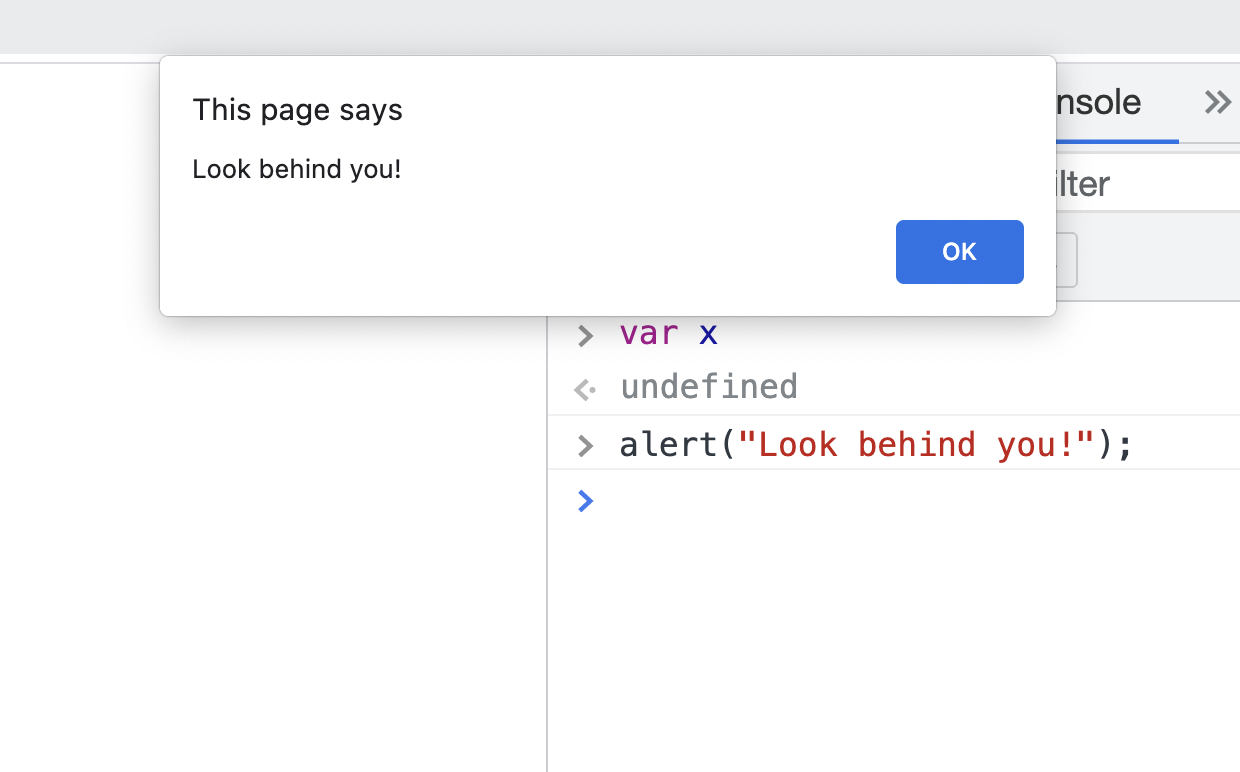
Just has the “OK.”
What does it return?
JavaScript
Confirm
// window.confirm(question)
confirm("Are you sure you want to delete your Facebook?"); // (Yes)
var confirmed = confirm("Are you sure you want to delete your Facebook?"); // returns true or false based on user choice
if (confirmed) {
// permanantly delete your facebook account
}
This window method will present you with a message and give you the option to “Cancel” (exit) or “OK” (confirm).
The function will return a value of true or false, which you can use to make decisions.
(good place to add an example of recursion)
Confirm
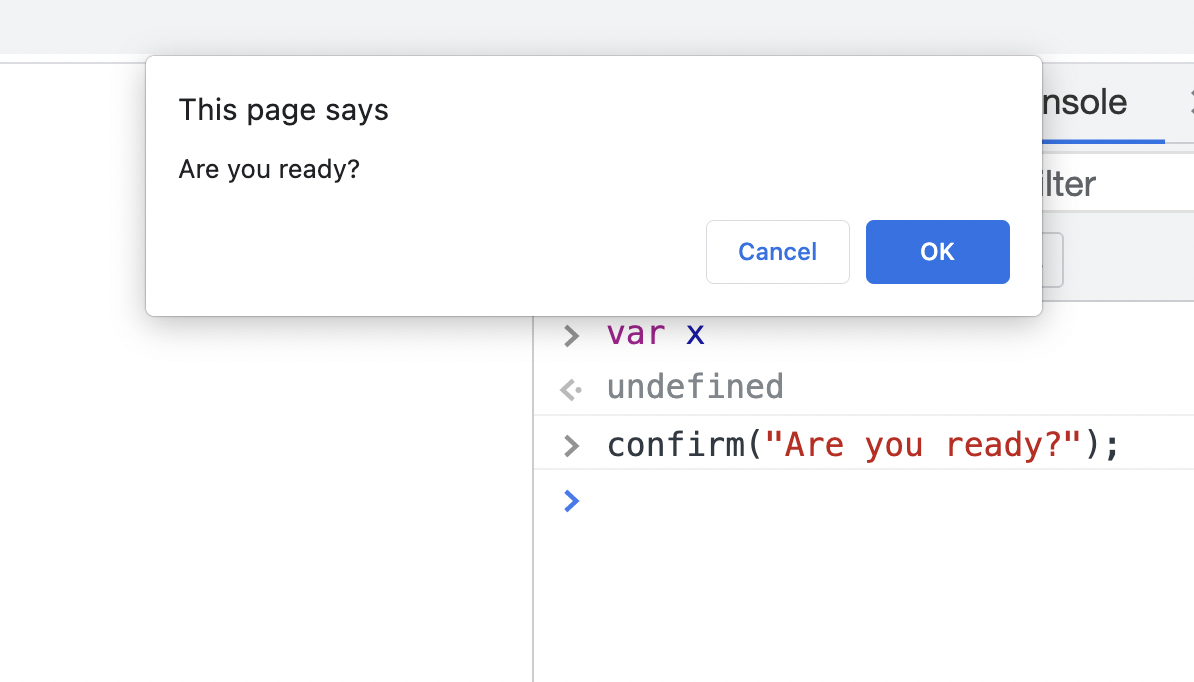
Basically a “yes” and “no.”
What does it return?
JavaScript
Prompt
// window.prompt(question)
prompt("What is your favorite color?"); // "Red"
var favoriteColor = prompt("What is your favorite color?"); // returns false or the value given (as a string)
console.log('color: ', favoriteColor);
if (favoriteColor == "Red") {
// yes?
} else {
// no?
}
Prompt
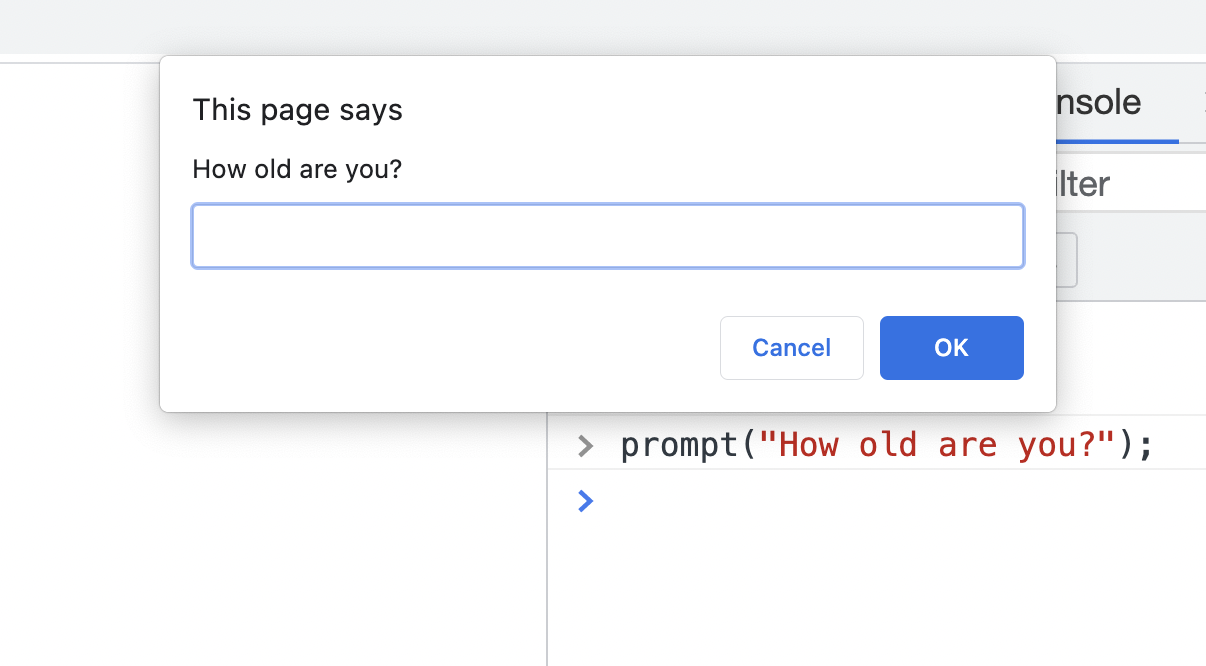
Now you have the “OK” and “Cancel” – but also a text input.
What does it return?
What can you put in there?
Exercises
-
Watch/follow along with the video
This will be enough to get your feet wet, but of course, you’ll need lots more practice.
-
Write out the pseudo code for each of the first 10 exercises for programmers
If you’ve already done this, then – do it again. Start over. Write out the human-readable steps. (just do the basic exercise – and not the challenges)
-
Write the code for the first 10 exercises for programmers
Set the timer. Stick to the most basic exercise requirements. You either accomplish them or you don’t. Get as many done as time allows. Post the code for all of them.
Optional reading
…
No!
Keep practicing today’s lesson.
Do it AGAIN!!!
and then again… until you are so bored – and fast / that you come up with an idea.